设计一个旅游景点导游模拟程序,为来访的客人提供景点最短路径的信息查询服务,任意选取n城市,构成一个有向带权图,图中顶点表示城市,边上的权值表示两点间的距离,根据用户指定的始点和终点输出相应的最短路径。咨询以用户和计算机的对话方式进行,由用户输入起始点和终点,输出信息:最短路径、并指出所经过的城市。存储结构选用邻接矩阵。使用c++语言,并添加菜单选项
时间: 2024-04-21 19:26:08 浏览: 132
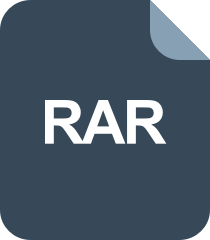
C++课程设计 旅游信息咨询程序
好的,我可以帮你设计旅游景点导游模拟程序。以下是程序的基本框架:
1. 定义一个City类,存储城市的名称和编号。
2. 定义一个Graph类,存储有向带权图的邻接矩阵,以及提供最短路径查询的方法。
3. 在程序中添加菜单选项,包括添加城市、添加路径、查询最短路径等功能。
4. 通过与用户的对话方式,获取用户输入的始点和终点,输出相应的最短路径信息。
下面是代码示例:
```c++
#include <iostream>
#include <vector>
#include <string>
#include <algorithm>
#include <queue>
using namespace std;
class City {
public:
City(string name, int id) : name(name), id(id) {}
string name;
int id;
};
class Graph {
public:
Graph(int n) : n(n), dist(n, vector<int>(n, INT_MAX)), path(n, vector<int>(n, -1)) {}
void addCity(string name) {
cities.push_back(City(name, cities.size()));
}
void addPath(int from, int to, int weight) {
dist[from][to] = weight;
path[from][to] = to;
}
void floyd() {
for (int k = 0; k < n; ++k) {
for (int i = 0; i < n; ++i) {
for (int j = 0; j < n; ++j) {
if (dist[i][k] != INT_MAX && dist[k][j] != INT_MAX && dist[i][j] > dist[i][k] + dist[k][j]) {
dist[i][j] = dist[i][k] + dist[k][j];
path[i][j] = path[i][k];
}
}
}
}
}
void printPath(int from, int to) {
cout << "最短路径长度:" << dist[from][to] << endl;
cout << "经过的城市:";
int cur = from;
while (cur != to) {
cout << cities[cur].name << " -> ";
cur = path[cur][to];
}
cout << cities[to].name << endl;
}
private:
vector<City> cities;
int n;
vector<vector<int>> dist;
vector<vector<int>> path;
};
int main() {
Graph graph(4);
graph.addCity("北京");
graph.addCity("上海");
graph.addCity("广州");
graph.addCity("深圳");
graph.addPath(0, 1, 1064);
graph.addPath(0, 2, 1162);
graph.addPath(0, 3, 1883);
graph.addPath(1, 0, 1064);
graph.addPath(1, 2, 1213);
graph.addPath(1, 3, 1703);
graph.addPath(2, 0, 1162);
graph.addPath(2, 1, 1213);
graph.addPath(2, 3, 1023);
graph.addPath(3, 0, 1883);
graph.addPath(3, 1, 1703);
graph.addPath(3, 2, 1023);
graph.floyd();
int from, to;
while (true) {
cout << "请选择功能:" << endl;
cout << "1. 查询最短路径" << endl;
cout << "2. 退出程序" << endl;
int choice;
cin >> choice;
if (choice == 1) {
cout << "请输入始发城市编号(0-3):" << endl;
cin >> from;
cout << "请输入终点城市编号(0-3):" << endl;
cin >> to;
graph.printPath(from, to);
} else if (choice == 2) {
break;
} else {
cout << "无效选择,请重新输入!" << endl;
}
}
return 0;
}
```
以上代码中,构造了一个有向带权图,其中4个城市分别用0-3的编号表示。使用Floyd算法求解最短路径,并通过菜单选项与用户进行交互,查询指定起点和终点之间的最短路径。
阅读全文
相关推荐
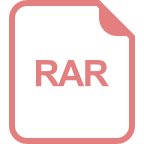


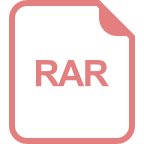
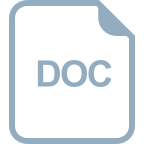
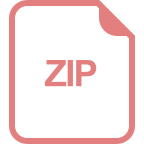
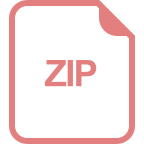
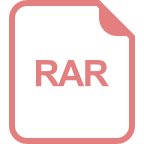
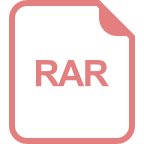
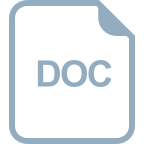
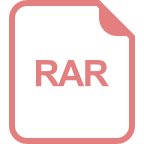
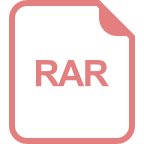
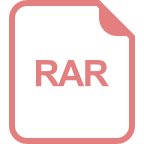
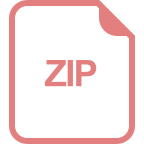
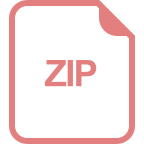
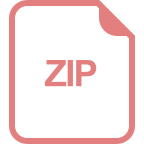