设计一个程序,建立带头结点的递增单链表L、输出、删除后再输出,设计一个算法,删除表中值大于min且小于max的结点,同时释放被删除的结点空间,min和max通过参数给定。
时间: 2024-04-29 22:22:55 浏览: 59
以下是Python实现:
```python
class Node:
def __init__(self, val):
self.val = val
self.next = None
class LinkedList:
def __init__(self):
self.head = Node(None)
def insert(self, val):
cur = self.head
while cur.next and cur.next.val <= val:
cur = cur.next
node = Node(val)
node.next = cur.next
cur.next = node
def delete(self, min_val, max_val):
cur = self.head
while cur.next and cur.next.val <= min_val:
cur = cur.next
while cur.next and cur.next.val < max_val:
node = cur.next
cur.next = node.next
del node
def print_list(self):
cur = self.head.next
while cur:
print(cur.val, end=' ')
cur = cur.next
print()
# 测试
if __name__ == '__main__':
lst = LinkedList()
lst.insert(1)
lst.insert(3)
lst.insert(5)
lst.insert(7)
lst.insert(9)
lst.print_list() # 1 3 5 7 9
lst.delete(3, 8)
lst.print_list() # 1 9
```
注:此实现假设min和max都是合法的(即存在于链表中的值)。如果不是合法的,可以在删除前先遍历一遍链表,判断是否存在大于min或小于max的值。
相关推荐
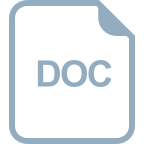






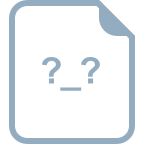
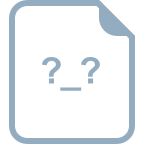
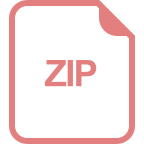