wordcount c语言实现
时间: 2023-10-10 22:13:03 浏览: 116
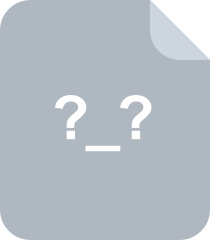
WordCount.c
下面是一个简单的 C 语言程序,用于统计给定文本中每个单词出现的次数:
```c
#include <stdio.h>
#include <string.h>
#include <ctype.h>
#define MAX_LEN 1000
#define MAX_WORDS 100
int main() {
char sentence[MAX_LEN];
char words[MAX_WORDS][MAX_LEN];
int freq[MAX_WORDS] = {0};
int i, j, count = 0;
printf("Enter a sentence: ");
fgets(sentence, MAX_LEN, stdin);
// 将字符串转换为小写字母,便于比较
for (i = 0; i < strlen(sentence); i++) {
sentence[i] = tolower(sentence[i]);
}
// 分离单词并统计词频
char *token = strtok(sentence, " ,.?!:;\n\r");
while (token != NULL) {
int found = 0;
for (i = 0; i < count; i++) {
if (strcmp(words[i], token) == 0) {
freq[i]++;
found = 1;
break;
}
}
if (!found) {
strcpy(words[count], token);
freq[count]++;
count++;
}
token = strtok(NULL, " ,.?!:;\n\r");
}
// 输出结果
for (i = 0; i < count; i++) {
printf("%s: %d\n", words[i], freq[i]);
}
return 0;
}
```
该程序首先要求用户输入一个字符串,然后将字符串中的所有单词转换为小写字母,以便于比较。接着使用 `strtok()` 函数将字符串分离成单独的单词,并统计每个单词出现的次数。最后输出每个单词及其出现次数。
阅读全文
相关推荐
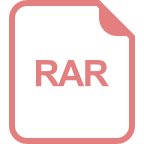

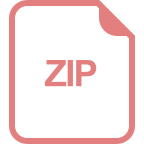
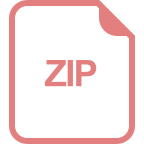

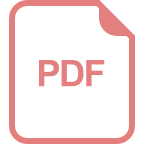
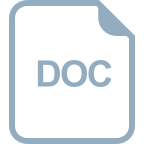
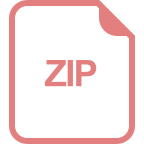
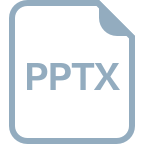
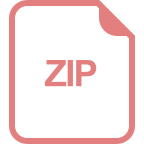
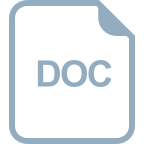




