ListInsert(SqList &L, int i, ElemType e)——在L中的第i个位置之前插入新的数据元素e,1≤i≤L.length+1。
时间: 2024-04-29 22:21:11 浏览: 11
The ListInsert operation is used to insert an element into a sequential list at a specified position. It takes the following parameters:
- SqList: The sequential list in which the element is to be inserted.
- i: The position at which the element is to be inserted.
- e: The element to be inserted.
The algorithm for ListInsert is as follows:
1. Check if the list is full. If it is, return an error.
2. Check if the position i is valid. If it is not, return an error.
3. Shift all elements from position i to the end of the list to the right by one position.
4. Insert the element e at position i.
5. Increment the length of the list by one.
Here is an implementation of the ListInsert operation in Python:
```
def ListInsert(SqList, i, e):
if len(SqList) == max_size:
return "Error: List is full"
if i < 1 or i > len(SqList) + 1:
return "Error: Invalid position"
for j in range(len(SqList), i - 1, -1):
SqList[j] = SqList[j - 1]
SqList[i - 1] = e
return SqList
```
This implementation assumes that the maximum size of the list is already defined as `max_size`. It returns an error message if the list is full or the position is invalid. Otherwise, it shifts the elements to the right and inserts the element at the specified position. It returns the updated list.
相关推荐
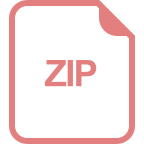
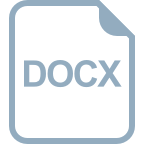
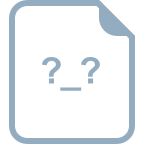















