·设顺序表L是递增有序表,试写一算法,将e插入到L中并使L仍是递增有序表。 • Void InsertList_Sq(SqList &L, Elemtype. e)求可实行的代码
时间: 2024-10-14 19:11:49 浏览: 45
要在顺序表(`SqList`)`L`中插入元素`e`并保持递增排序,你可以使用以下步骤编写一个简单的算法:
1. 检查列表是否为空或最后一个元素小于新值`e`,如果是,则直接将`e`添加为第一个元素。
2. 遍历已排序的列表,找到正确的位置`i`,使得`L[i] <= e < L[i+1]`。
3. 将`e`插入到位置`i+1`。
以下是对应的C语言代码实现:
```c
#include <stdio.h>
// 假设 SqList 是一个结构体,包含指针 head 和 length
typedef struct {
void* data;
struct SqList* next;
} SqListNode;
struct SqList {
SqListNode* head;
int length;
};
void insertInSorted(SqList* L, void* e) {
if (L->length == 0 || *(int*)L->head->data < *(int*)e) { // 如果列表为空或新元素比当前最小大
// 创建一个新的节点并将其设置为头结点
SqListNode newNode = {e, NULL};
L->head = &newNode;
L->length++;
return;
}
// 找到插入位置
SqListNode* current = L->head;
for (int i = 1; i < L->length && *(int*)current->next->data < *(int*)e; ++i, current = current->next);
// 插入新元素
current->next = (SqListNode*)malloc(sizeof(SqListNode));
current->next->data = e;
current->next->next = current->next->next;
L->length++;
}
// 示例用法
int main() {
SqList list;
list.head = NULL;
list.length = 0;
// 初始化和插入元素
insertInSorted(&list, 5); // 插入5
insertInSorted(&list, 3); // 插入3
insertInSorted(&list, 7); // 插入7
// 打印结果
SqListNode* temp = list.head;
while (temp != NULL) {
printf("%d ", *(int*)temp->data);
temp = temp->next;
}
printf("\n");
return 0;
}
```
阅读全文
相关推荐
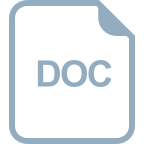
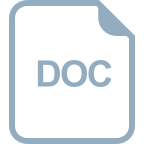
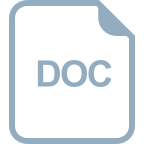















