设顺序表L是递增有序表,利用C语言试写一算法,将x插入到L中并使L仍是递增有序表
时间: 2024-09-21 15:04:41 浏览: 42
在C语言中,如果你想将元素x插入一个已经递增有序的顺序表L中,可以采用二分查找法找到合适的位置,然后插入新元素。下面是一个简单的插入算法示例:
```c
#include <stdio.h>
// 假设顺序表L存储整数,每个元素占用一个int类型的空间
typedef struct {
int data;
struct Node* next; // 指向下一个元素的指针
} Node;
void insert(int x, Node** head) { // 参数head指向当前头节点的指针
if (*head == NULL || x <= (*head)->data) { // 如果表为空或x小于等于头元素
(*head) = (Node*)malloc(sizeof(Node)); // 插入新元素作为第一个元素
(*head)->data = x;
(*head)->next = NULL;
} else { // 否则从第二个元素开始查找插入位置
Node* current = *head->next;
while (current != NULL && x > current->data) {
current = current->next;
}
if (current == NULL) { // 如果x大于链表中的所有元素,插入到最后
current = *head;
while (current->next != NULL) {
current = current->next;
}
current->next = (Node*)malloc(sizeof(Node));
current->next->data = x;
current->next->next = NULL;
} else { // 插入到找到的位置
Node* newNode = (Node*)malloc(sizeof(Node));
newNode->data = x;
newNode->next = current->next;
current->next = newNode;
}
}
}
// 示例如何使用上述函数插入元素
int main() {
Node* head = NULL; // 初始化空链表
// 插入元素
insert(10, &head); // 第一次插入
insert(20, &head); // 插入20
insert(15, &head); // 插入15
// 打印链表验证结果
Node* temp = head;
while (temp != NULL) {
printf("%d -> ", temp->data);
temp = temp->next;
}
printf("NULL\n");
return 0;
}
阅读全文
相关推荐
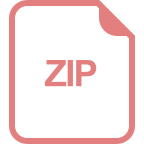
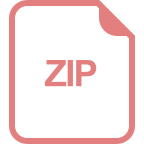
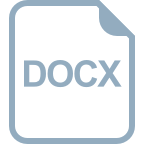















