顺序表是一个递增顺序表,写一个算法,将x插入L后,L仍为一个有序表(c语言)
时间: 2024-09-25 07:10:36 浏览: 44
在C语言中,如果你有一个递增的顺序表(升序排列),并且想要将一个数`x`插入到列表`L`之后保持有序,可以使用二分查找法找到`x`应该插入的位置,然后像前面所述的步骤一样插入元素。这里提供了一个简单的算法实现:
```c
#include <stdio.h>
// 假设顺序表L的头指针、元素类型以及长度
typedef struct Node {
int data;
struct Node* next;
} Node;
// 二分查找函数,返回x应该插入的位置(索引)
int binary_search(Node* L, int x, int low, int high) {
while (low <= high) {
int mid = (low + high) / 2;
if (L[mid].data < x) {
low = mid + 1;
} else {
high = mid - 1;
}
}
return low; // 返回插入位置(注意不是索引加一)
}
// 插入函数
void insert_sorted(Node** L, int x) {
int position = binary_search(*L, x, 0, (*L)->length - 1);
// 分配新的内存
Node* newNode = (Node*)malloc(sizeof(Node));
newNode->data = x;
newNode->next = NULL;
// 将newNode插入到合适位置
if (position == 0) { // 如果x是最小值,插入头部
newNode->next = *L;
*L = newNode;
} else {
Node* current = *L;
for (int i = 0; i < position - 1; i++) {
current = current->next;
}
newNode->next = current->next;
current->next = newNode;
}
}
// 示例使用
void print_list(Node* L) {
while (L != NULL) {
printf("%d -> ", L->data);
L = L->next;
}
printf("NULL\n");
}
int main() {
// 初始化顺序表...
Node* L = ...; // 例如 {1, 3, 5, 7, 9}
int x = 6; // 要插入的数值
insert_sorted(&L, x);
printf("After insertion, the list is:\n");
print_list(L);
return 0;
}
```
阅读全文
相关推荐
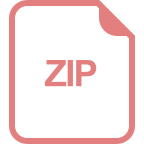
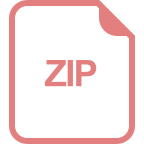
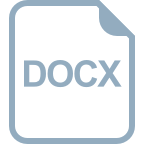















