设顺序表L是递增有序表,试写一算法,将插入到L中并使L仍是递增有序表。请给出完整的C程序并上机调试通过。
时间: 2024-09-20 12:04:26 浏览: 44
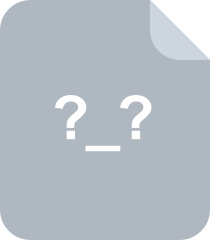
数据结构与算法c++实现 两个顺序表的元素严格单调递增,将其共同元素保存到新顺序表中,且为递增排序

在C语言中,我们可以创建一个简单的函数来插入一个元素到一个递增有序列表`L`中,并保持其递增顺序。这里是一个基本的示例:
```c
#include <stdio.h>
// 定义链表节点结构
typedef struct Node {
int data;
struct Node* next;
} Node;
// 插入函数,保持递增顺序
void insert(int value, Node** head) {
// 如果链表为空或者新值小于当前头节点,直接作为新的头节点
if (*head == NULL || value <= (*head)->data) {
Node* newNode = (Node*)malloc(sizeof(Node));
newNode->data = value;
newNode->next = *head;
*head = newNode;
return;
}
// 遍历查找合适的位置插入
for (Node* current = *head; current->next != NULL && value > current->next->data; current = current->next) {}
// 在找到合适位置或者到达链表末尾时插入新节点
if (current->next == NULL) { // 到达链表末尾
Node* newNode = (Node*)malloc(sizeof(Node));
newNode->data = value;
newNode->next = NULL;
current->next = newNode;
} else { // 找到插入点
newNode = (Node*)malloc(sizeof(Node));
newNode->data = value;
newNode->next = current->next;
current->next = newNode;
}
}
int main() {
// 初始化空链表
Node* head = NULL;
// 测试插入操作
insert(5, &head); // L = [5]
insert(3, &head); // L = [3, 5]
insert(8, &head); // L = [3, 5, 8]
insert(1, &head); // L = [1, 3, 5, 8]
// 输出链表检查结果(这里只是一个演示,实际项目中可能需要其他方式遍历)
for (Node* node = head; node != NULL; node = node->next) {
printf("%d ", node->data);
}
printf("\n");
return 0;
}
阅读全文
相关推荐
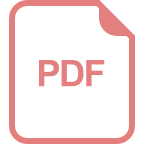















