python矩阵变换
时间: 2024-01-07 21:23:51 浏览: 196
以下是一些常见的Python矩阵变换操作:
1. 转换矩阵类型:
```python
import numpy as np
# 将列表转换为矩阵
list1 = [[1, 2, 3], [4, 5, 6]]
matrix1 = np.array(list1)
# 将矩阵转换为列表
list2 = matrix1.tolist()
```
2. 矩阵维度增减:
```python
import numpy as np
# 增加维度
matrix1 = np.array([[1, 2, 3], [4, 5, 6]])
matrix2 = np.expand_dims(matrix1, axis=0) # 在第0维度上增加维度
# 减少维度
matrix3 = np.squeeze(matrix2, axis=0) # 在第0维度上减少维度
```
3. 多矩阵拼接:
```python
import numpy as np
# 水平拼接
matrix1 = np.array([[1, 2], [3, 4]])
matrix2 = np.array([[5, 6]])
result1 = np.hstack((matrix1, matrix2))
# 垂直拼接
matrix3 = np.array([[7, 8]])
result2 = np.vstack((matrix1, matrix3))
```
4. 矩阵切片获取:
```python
import numpy as np
matrix1 = np.array([[1, 2, 3], [4, 5, 6], [7, 8, 9]])
# 获取第一行
row1 = matrix1[0, :]
# 获取第一列
column1 = matrix1[:, 0]
# 获取子矩阵
submatrix = matrix1[1:3, 1:3]
```
5. 多矩阵迭代:
```python
import numpy as np
matrix1 = np.array([[1, 2], [3, 4]])
# 迭代行
for row in matrix1:
print(row)
# 迭代列
for column in matrix1.T:
print(column)
```
阅读全文
相关推荐
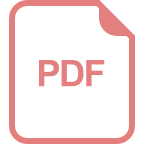
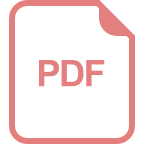
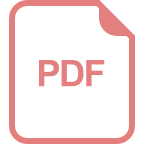















