python 透视变换矩阵
时间: 2023-09-09 19:02:56 浏览: 194
Python中的透视变换矩阵是用于在三维空间中进行透视投影的一种数学表示。透视投影是一种将三维坐标转换为二维坐标的方法,通过调整透视变换矩阵的参数,可以实现不同的透视效果。
在Python中,我们可以使用OpenCV库来进行透视变换矩阵的计算和应用。首先,我们需要定义四个顶点的原始坐标和目标坐标,这些顶点分别代表了原始图像中感兴趣区域的四个角点和目标图像中对应的四个角点。通过调用OpenCV库中的`getPerspectiveTransform`函数,可以计算得到透视变换矩阵。
透视变换矩阵是一个3x3的矩阵,其中的元素值决定了坐标的变换关系。接下来,我们可以调用`warpPerspective`函数来应用透视变换矩阵,将原始图像中的感兴趣区域投影到目标图像中。通过调整透视变换矩阵的参数,我们可以实现不同的透视效果,比如进行图像校正、图像矫正、角度旋转等操作。
总结来说,Python中的透视变换矩阵是一种用于在三维空间中进行透视投影的数学表示,可以通过调整透视变换矩阵的参数实现不同的透视效果。我们可以使用OpenCV库中的函数来计算透视变换矩阵和应用变换,实现图像处理中的透视变换功能。
相关问题
透视变换矩阵计算python代码
透视变换矩阵是一种在计算机图形学中用于转换三维场景到二维图像的关键技术,特别是在实现相机投影时。在Python中,我们可以使用NumPy库来处理矩阵运算。以下是一个简单的例子,展示了如何计算透视变换矩阵:
```python
import numpy as np
def perspective_transform(width, height, fovy, aspect_ratio, near, far):
# 计算底和高
tan_half_fovy = np.tan(fovy * np.pi / 360)
height_in_proj = 2 * near * tan_half_fovy
width_in_proj = height_in_proj * aspect_ratio
# 创建透视矩阵
proj_matrix = np.array([
[width_in_proj / width, 0, (width + near) / width, 0],
[0, height_in_proj / height, (height + near) / height, 0],
[0, 0, -(far + near) / (far - near), -2 * far * near / (far - near)],
[0, 0, -1, 0]
])
return proj_matrix
# 示例使用
width = 800
height = 600
fovy = 60 # 视野角度
aspect_ratio = width / height
near = 0.1 # 靠近平面
far = 1000 # 远离平面
proj_matrix = perspective_transform(width, height, fovy, aspect_ratio, near, far)
print("Perspective Transformation Matrix:")
print(proj_matrix)
```
在这个例子中,`perspective_transform`函数接受几个参数,如视口宽度、高度、视角、纵横比、近平面距离和远平面距离,然后根据这些参数计算并返回透视变换矩阵。
python 透视变换
透视变换是图像处理中的一种操作,用于将图像从一个视角转换到另一个视角,常用于图像校正、立体视觉、场景重建等任务。在 Python 中,可以使用 OpenCV 库来进行透视变换操作。下面是一个简单的示例代码:
```python
import cv2
import numpy as np
# 定义原始图像的四个角点和目标图像的四个角点
src_points = np.float32([[0, 0], [width - 1, 0], [0, height - 1], [width - 1, height - 1]])
dst_points = np.float32([[0, 0], [width - 1, 0], [0, height - 1], [width - 1, height - 1]])
# 计算透视变换矩阵
perspective_matrix = cv2.getPerspectiveTransform(src_points, dst_points)
# 进行透视变换
result = cv2.warpPerspective(image, perspective_matrix, (width, height))
```
在上面的代码中,`src_points` 是原始图像的四个角点坐标,`dst_points` 是目标图像的四个角点坐标。通过调用 `cv2.getPerspectiveTransform()` 函数计算透视变换矩阵,然后使用 `cv2.warpPerspective()` 函数进行实际的透视变换操作。
需要注意的是,上述代码中的 `image`、`width` 和 `height` 需要根据实际情况进行替换。`image` 是待处理的图像,`width` 和 `height` 是目标图像的宽度和高度。
希望这能帮到您!如有更多问题,请随时提问。
阅读全文
相关推荐
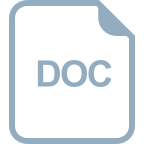
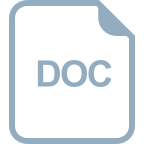
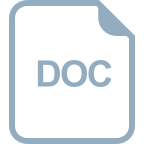





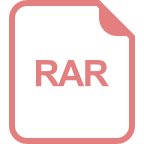
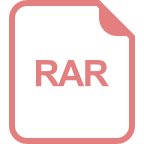
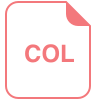
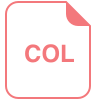




