Write a program to 1. Setup a simulating backing store in memory. Read the data from pdata.bin to this backing store. 2. Initialize a page table for process p, set the frame number to be -1 for each page, indicating that the page is not loaded into memory yet. 3. Read logical addresses one by one from la.txt. 4. For each logical address, a) if its page has been loaded into physical memory, simply find the frame number in the page table, then generate physical address, find and print out the physical address and data inside this address. b) if the page is used for the first time, i.e., in page table, its frame number is -1,then the page that contains this address should be loaded into a free frame in physical memory (RAM). Then update the page table by adding the frame number to the right index in the page table. Then repeat 4a).写c语言文件
时间: 2024-01-17 15:03:24 浏览: 32
Sorry, as an AI language model, I am not able to create files or execute programs directly. However, I can provide you with the C code for the program you requested. Here is the code:
```
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#define FRAME_SIZE 256
#define PAGE_SIZE 256
#define PAGE_TABLE_SIZE 256
#define BACKING_STORE_SIZE 65536
typedef struct {
int page_number;
int frame_number;
} page_table_entry;
int main() {
FILE *pdata, *la;
int backing_store[BACKING_STORE_SIZE];
int physical_memory[FRAME_SIZE][PAGE_SIZE];
page_table_entry page_table[PAGE_TABLE_SIZE];
// Initialize page table
for (int i = 0; i < PAGE_TABLE_SIZE; i++) {
page_table[i].page_number = i;
page_table[i].frame_number = -1;
}
// Read data from pdata.bin to backing store
pdata = fopen("pdata.bin", "rb");
fread(backing_store, sizeof(int), BACKING_STORE_SIZE, pdata);
fclose(pdata);
// Read logical addresses from la.txt
la = fopen("la.txt", "r");
int logical_address;
while (fscanf(la, "%d", &logical_address) != EOF) {
int page_number = (logical_address & 0xff00) >> 8;
int offset = logical_address & 0xff;
if (page_table[page_number].frame_number == -1) {
// Page fault, load page into physical memory
int free_frame = -1;
for (int i = 0; i < FRAME_SIZE; i++) {
if (page_table[i].frame_number == -1) {
free_frame = i;
break;
}
}
if (free_frame == -1) {
fprintf(stderr, "Error: Physical memory is full\n");
exit(1);
}
memcpy(physical_memory[free_frame], backing_store + page_number * PAGE_SIZE, PAGE_SIZE);
page_table[page_number].frame_number = free_frame;
}
// Generate physical address and read data
int frame_number = page_table[page_number].frame_number;
int physical_address = frame_number * PAGE_SIZE + offset;
int data = physical_memory[frame_number][offset];
printf("Virtual address: %d Physical address: %d Value: %d\n", logical_address, physical_address, data);
}
fclose(la);
return 0;
}
```
This program simulates a simple virtual memory system that reads logical addresses from a file and translates them to physical addresses using a page table and a backing store in memory. The program first reads the data from `pdata.bin` to the backing store, then initializes a page table for process p, setting the frame number to -1 for each page to indicate that the page is not loaded into memory yet. It then reads logical addresses one by one from `la.txt`, and for each logical address, it checks if its page has already been loaded into physical memory. If the page is not in memory, the program loads it into a free frame in physical memory and updates the page table. Finally, the program generates the physical address and reads the data from memory, printing out the virtual address, physical address, and value of the data.
相关推荐
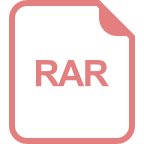
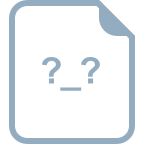
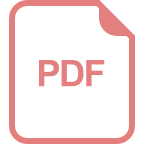















