C语言实现 串口读 并支持115200和1500000等多个波特率,请写出c语言串口程序
时间: 2024-03-05 11:52:25 浏览: 148
好的,以下是一个简单的 C 语言程序,用于读取串口数据并支持多个波特率:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <unistd.h>
#include <fcntl.h>
#include <termios.h>
// 串口设备文件名
#define DEVICE "/dev/ttyS0"
int main(int argc, char *argv[])
{
int fd;
char buf[256];
int baudrate = B115200; // 默认波特率为 115200
// 如果指定了波特率参数,则使用指定的波特率
if (argc > 1) {
if (strcmp(argv[1], "115200") == 0) {
baudrate = B115200;
} else if (strcmp(argv[1], "1500000") == 0) {
baudrate = B1500000;
}
}
// 打开串口设备文件
fd = open(DEVICE, O_RDWR | O_NOCTTY);
if (fd < 0) {
perror("open");
exit(1);
}
// 配置串口属性
struct termios options;
tcgetattr(fd, &options);
cfsetispeed(&options, baudrate);
cfsetospeed(&options, baudrate);
options.c_cflag |= (CLOCAL | CREAD);
options.c_cflag &= ~PARENB;
options.c_cflag &= ~CSTOPB;
options.c_cflag &= ~CSIZE;
options.c_cflag |= CS8;
options.c_lflag &= ~(ICANON | ECHO | ECHOE | ISIG);
options.c_iflag &= ~(IXON | IXOFF | IXANY);
options.c_oflag &= ~OPOST;
options.c_cc[VMIN] = 1;
options.c_cc[VTIME] = 0;
tcsetattr(fd, TCSANOW, &options);
// 读取串口数据
while (1) {
int len = read(fd, buf, sizeof(buf));
if (len > 0) {
buf[len] = 0;
printf("%s", buf);
}
}
close(fd);
return 0;
}
```
这个程序使用了 Linux 下的串口设备文件 `/dev/ttyS0`,你需要根据实际情况修改这个文件名。如果你使用的是 Windows 系统,则需要使用 `CreateFile`、`ReadFile` 等 Win32 API 来实现串口读取。另外,本程序只是一个示例,如果你需要更加丰富的功能,比如写入数据到串口等,可以自己进行扩展。
阅读全文
相关推荐
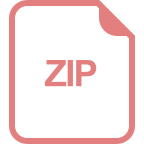
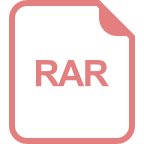
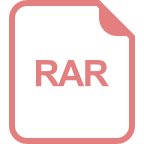















