linux c Select和termios有什么区别,请详细解释,并写一个分别使用select的c语言程序和termios的c语言程序,使用串口
时间: 2024-02-24 21:58:43 浏览: 160
`select`和`termios`都是Linux下用于串口编程的常见工具,但它们的作用不同。
`select`是一种多路复用机制,用于同时监控多个文件描述符的状态,以便在有数据可读、可写或错误发生时立即对其进行操作。在串口编程中,`select`可以用于同时监听多个串口的数据,以便对它们进行处理。
`termios`是一种用于配置串口通信参数的API。通过`termios`,可以设置串口的波特率、数据位、停止位、奇偶校验等参数,以便与其他设备进行通信。
下面是使用`select`的C语言程序和使用`termios`的C语言程序,分别使用串口进行数据的读取:
使用`select`的C语言程序:
```c
#include <stdio.h>
#include <stdlib.h>
#include <fcntl.h>
#include <unistd.h>
#include <termios.h>
#include <sys/select.h>
int main() {
int fd1, fd2;
char buf[1024];
fd_set input_fd_set;
// 打开串口1
fd1 = open("/dev/ttyUSB0", O_RDWR | O_NOCTTY | O_NONBLOCK);
if (fd1 < 0) {
printf("Open ttyUSB0 failed!\n");
exit(1);
}
// 打开串口2
fd2 = open("/dev/ttyUSB1", O_RDWR | O_NOCTTY | O_NONBLOCK);
if (fd2 < 0) {
printf("Open ttyUSB1 failed!\n");
exit(1);
}
// 配置串口1参数
struct termios options1;
tcgetattr(fd1, &options1);
cfsetispeed(&options1, B9600);
cfsetospeed(&options1, B9600);
options1.c_cflag |= (CLOCAL | CREAD);
options1.c_cflag &= ~PARENB;
options1.c_cflag &= ~CSTOPB;
options1.c_cflag &= ~CSIZE;
options1.c_cflag |= CS8;
tcsetattr(fd1, TCSANOW, &options1);
// 配置串口2参数
struct termios options2;
tcgetattr(fd2, &options2);
cfsetispeed(&options2, B9600);
cfsetospeed(&options2, B9600);
options2.c_cflag |= (CLOCAL | CREAD);
options2.c_cflag &= ~PARENB;
options2.c_cflag &= ~CSTOPB;
options2.c_cflag &= ~CSIZE;
options2.c_cflag |= CS8;
tcsetattr(fd2, TCSANOW, &options2);
while (1) {
// 监听串口1和串口2的数据
FD_ZERO(&input_fd_set);
FD_SET(fd1, &input_fd_set);
FD_SET(fd2, &input_fd_set);
select(FD_SETSIZE, &input_fd_set, NULL, NULL, NULL);
// 读取串口1的数据
if (FD_ISSET(fd1, &input_fd_set)) {
int n = read(fd1, buf, 1024);
if (n > 0) {
printf("Read from ttyUSB0: %s\n", buf);
}
}
// 读取串口2的数据
if (FD_ISSET(fd2, &input_fd_set)) {
int n = read(fd2, buf, 1024);
if (n > 0) {
printf("Read from ttyUSB1: %s\n", buf);
}
}
}
return 0;
}
```
使用`termios`的C语言程序:
```c
#include <stdio.h>
#include <stdlib.h>
#include <fcntl.h>
#include <unistd.h>
#include <termios.h>
int main() {
int fd;
char buf[1024];
// 打开串口
fd = open("/dev/ttyUSB0", O_RDWR | O_NOCTTY);
if (fd < 0) {
printf("Open ttyUSB0 failed!\n");
exit(1);
}
// 配置串口参数
struct termios options;
tcgetattr(fd, &options);
cfsetispeed(&options, B9600);
cfsetospeed(&options, B9600);
options.c_cflag |= (CLOCAL | CREAD);
options.c_cflag &= ~PARENB;
options.c_cflag &= ~CSTOPB;
options.c_cflag &= ~CSIZE;
options.c_cflag |= CS8;
tcsetattr(fd, TCSANOW, &options);
while (1) {
// 读取串口数据
int n = read(fd, buf, 1024);
if (n > 0) {
printf("Read from ttyUSB0: %s\n", buf);
}
}
return 0;
}
```
需要注意的是,以上代码仅作为示例,实际使用时需要根据具体的需求进行修改和优化。
阅读全文
相关推荐
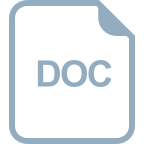
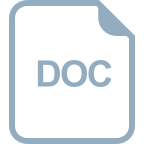
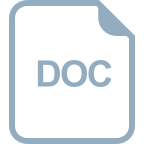
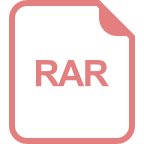




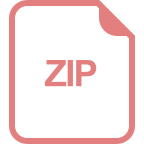
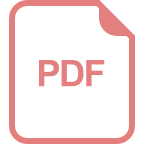
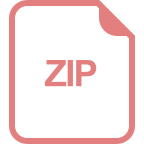
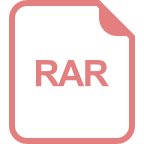
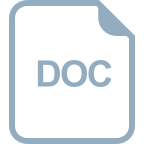
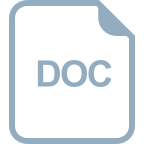
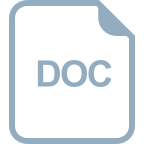
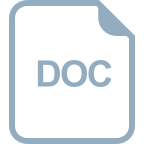
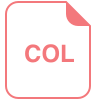
