写一段STM32F412的ADC DMA乒乓buffer的代码
时间: 2023-12-01 21:00:04 浏览: 116
以下是一个简单的STM32F412的ADC DMA乒乓buffer的代码,用于连续读取ADC采样数据并将其存储在两个不同的缓冲区中:
```c
#define BUFFER_SIZE 256
uint16_t bufferA[BUFFER_SIZE];
uint16_t bufferB[BUFFER_SIZE];
uint16_t *currentBuffer = bufferA;
void initADC_DMA(void) {
// Enable ADC clock
RCC->APB2ENR |= RCC_APB2ENR_ADC1EN;
// Enable DMA clock
RCC->AHB1ENR |= RCC_AHB1ENR_DMA2EN;
// Configure ADC1
ADC1->CR2 = ADC_CR2_ADON; // Turn on ADC
ADC1->CR2 |= ADC_CR2_CONT; // Enable continuous conversion mode
ADC1->SQR1 = 0; // Only one conversion
ADC1->SQR3 = ADC_CHANNEL_0; // Use channel 0
// Configure DMA2 channel 0
DMA2_Stream0->CR = 0; // Disable DMA channel
DMA2_Stream0->CR = DMA_SxCR_CHSEL_0 | DMA_SxCR_CHSEL_1 | DMA_SxCR_CHSEL_2; // Select ADC1 channel 0
DMA2_Stream0->CR |= DMA_SxCR_PL_1; // Set DMA priority to high
DMA2_Stream0->CR |= DMA_SxCR_MSIZE_0; // Set DMA memory size to 16 bits
DMA2_Stream0->CR |= DMA_SxCR_PSIZE_0; // Set DMA peripheral size to 16 bits
DMA2_Stream0->CR |= DMA_SxCR_MINC; // Enable memory increment mode
DMA2_Stream0->CR |= DMA_SxCR_CIRC; // Enable circular mode
DMA2_Stream0->CR |= DMA_SxCR_DBM; // Enable double buffer mode
DMA2_Stream0->NDTR = BUFFER_SIZE; // Set buffer size
DMA2_Stream0->M0AR = (uint32_t)bufferA; // Set buffer A address
DMA2_Stream0->M1AR = (uint32_t)bufferB; // Set buffer B address
DMA2_Stream0->PAR = (uint32_t)&(ADC1->DR); // Set ADC data register address
DMA2_Stream0->CR |= DMA_SxCR_TCIE; // Enable transfer complete interrupt
// Enable DMA channel
DMA2_Stream0->CR |= DMA_SxCR_EN;
// Start ADC conversion
ADC1->CR2 |= ADC_CR2_DMA; // Enable DMA mode
ADC1->CR2 |= ADC_CR2_SWSTART; // Start conversion
}
void DMA2_Stream0_IRQHandler(void) {
if (DMA2->LISR & DMA_LISR_TCIF0) {
DMA2->LIFCR |= DMA_LIFCR_CTCIF0; // Clear transfer complete flag
if (currentBuffer == bufferA) {
currentBuffer = bufferB;
} else {
currentBuffer = bufferA;
}
DMA2_Stream0->M0AR = (uint32_t)currentBuffer; // Update buffer address
}
}
int main(void) {
initADC_DMA();
while (1) {
// Do something with currentBuffer
}
return 0;
}
```
在上面的代码中,我们使用了DMA2的stream0来读取ADC采样数据,并使用乒乓buffer来处理连续的数据流。首先,在`initADC_DMA()`函数中,我们启用了ADC和DMA的时钟,并配置了ADC和DMA的寄存器。我们将ADC配置为连续转换模式,并使用通道0进行采样。然后,我们将DMA配置为使用ADC1的数据寄存器作为外设,将bufferA和bufferB作为内存,并启用了循环和双缓冲模式。
在中断处理函数`DMA2_Stream0_IRQHandler()`中,我们检查DMA的传输完成标志,并在每次传输完成时切换当前缓冲区。最后,在主函数中,我们可以使用`currentBuffer`指针来访问当前的缓冲区中的数据。
阅读全文
相关推荐
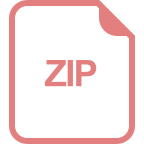
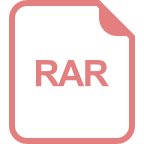
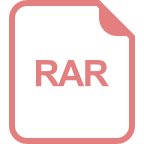




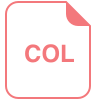
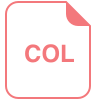
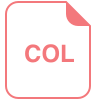
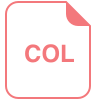
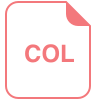
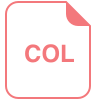
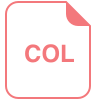
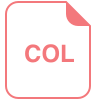
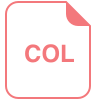
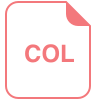