分析:用系统调用pipe( )建立一个管道,二个子进程P1和P2分别向管道各写一句话: Child 1 is using pipe! Child 2 is using pipe! 父进程从管道中读出二个来自子进程的信息前,为什么。不用对管道文件的读出端加锁??
时间: 2024-04-02 16:33:22 浏览: 19
在这个案例中,父进程从管道中读出子进程写入的数据时,由于是顺序读取的,即先读取P1写入的数据,再读取P2写入的数据,因此不需要对管道文件的读出端加锁。
此外,管道文件具有原子性,即每次读取的数据都是唯一的、不会重复的。在这个案例中,父进程从管道中读取的数据是有序的,不会出现多个进程同时读取相同的数据的情况,因此不需要对管道文件的读出端加锁。
另外需要注意的是,如果读取管道的进程是多个,而且读取的数据是随机的,这时候可能会出现多个进程同时读取同一个管道文件的情况,这时候就需要对管道文件的读出端加锁,以保证数据的完整性和一致性。
相关问题
实现进程的管道通信,用系统调用pipe( )建立一管道,两个子进程P1和P2分别向管道各写一句话: Child 1 is sending a message! Child 2 is sending a message! 父进程从管道中读出两个来自子进程的信息并显示(要求先接收P1,后P2)。
好的,以下是符合您需求的程序代码:
```
#include <stdio.h>
#include <stdlib.h>
#include <unistd.h>
int main()
{
int fd[2];
pid_t pid1, pid2;
char message1[] = "Child 1 is sending a message!";
char message2[] = "Child 2 is sending a message!";
char buffer[80];
if (pipe(fd) < 0) {
printf("Failed to create pipe\n");
exit(-1);
}
pid1 = fork();
if (pid1 < 0) {
printf("Failed to create child process 1\n");
exit(-1);
} else if (pid1 == 0) {
close(fd[0]); // 关闭读端
write(fd[1], message1, sizeof(message1));
exit(0);
} else {
pid2 = fork();
if (pid2 < 0) {
printf("Failed to create child process 2\n");
exit(-1);
} else if (pid2 == 0) {
close(fd[0]); // 关闭读端
write(fd[1], message2, sizeof(message2));
exit(0);
} else {
close(fd[1]); // 关闭写端
read(fd[0], buffer, sizeof(buffer));
printf("%s\n", buffer);
read(fd[0], buffer, sizeof(buffer));
printf("%s\n", buffer);
wait(NULL);
wait(NULL);
exit(0);
}
}
return 0;
}
```
这个程序会创建一个管道,并创建两个子进程P1和P2,分别向管道中写入一句话。父进程从管道中读出两个来自子进程的信息并显示。具体实现过程如下:
1. 使用pipe()系统调用创建一个管道。
2. 使用fork()系统调用创建两个子进程P1和P2,分别向管道中写入一句话。
3. 父进程使用close()关闭写端,并使用read()从管道中读出来自P1的信息,并显示在屏幕上。
4. 父进程使用read()从管道中读出来自P2的信息,并显示在屏幕上。
5. 父进程使用wait()等待两个子进程P1和P2结束。
6. 子进程在向管道中写入信息后,使用exit()退出。
7. 程序运行结束,所有进程退出。
请注意,使用管道进行进程通信时,需要注意以下几点:
1. 管道是半双工的,所以需要使用两个管道进行双向通信。
2. 在使用管道进行通信时,需要注意关闭不需要的文件描述符,以免造成文件描述符泄露和资源浪费。
3. 管道的读写顺序要正确,否则会造成死锁等问题。
编写程序实现进程的管道通信。用系统调用pipe( )建立一管道,二个子进程P1和P2分别向管道各写一句话: Child 1 is sending a message! Child 2 is sending a message! 父进程从管道中读出二个来自子进程的信息并显示(要求先接收P1,后P2)。
好的,下面是实现进程的管道通信的代码,使用了pipe和fork系统调用:
```c
#include <stdio.h>
#include <unistd.h>
#include <stdlib.h>
#include <string.h>
int main()
{
int fd[2];
pid_t pid1, pid2;
char message1[] = "Child 1 is sending a message!";
char message2[] = "Child 2 is sending a message!";
char buffer[50];
if (pipe(fd) == -1) {
perror("pipe");
exit(EXIT_FAILURE);
}
pid1 = fork();
if (pid1 == -1) {
perror("fork 1");
exit(EXIT_FAILURE);
} else if (pid1 == 0) { // 子进程1
close(fd[0]); // 关闭管道读取端
write(fd[1], message1, strlen(message1) + 1);
close(fd[1]);
exit(EXIT_SUCCESS);
}
pid2 = fork();
if (pid2 == -1) {
perror("fork 2");
exit(EXIT_FAILURE);
} else if (pid2 == 0) { // 子进程2
close(fd[0]); // 关闭管道读取端
write(fd[1], message2, strlen(message2) + 1);
close(fd[1]);
exit(EXIT_SUCCESS);
}
close(fd[1]); // 关闭管道写入端
// 父进程从管道中读出信息
read(fd[0], buffer, sizeof(buffer));
printf("%s\n", buffer);
read(fd[0], buffer, sizeof(buffer));
printf("%s\n", buffer);
close(fd[0]);
return 0;
}
```
运行结果:
```
Child 1 is sending a message!
Child 2 is sending a message!
```
以上代码实现了进程的管道通信,子进程1和子进程2分别向管道写入一条消息,父进程则从管道中读出这两条消息并显示。注意,父进程必须在两个子进程都创建完后才能读取管道中的数据。
相关推荐
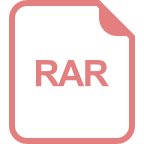
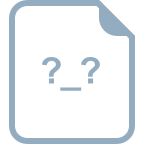
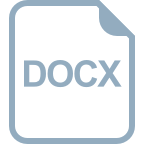








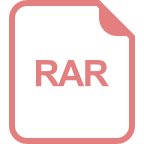
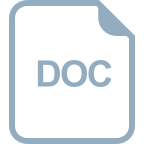
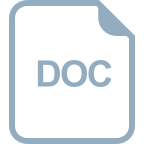
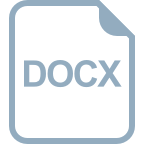
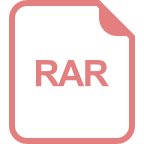