用python写一个kmeans程序
时间: 2023-03-19 07:23:00 浏览: 126
好的,下面是一个使用Python实现K均值聚类算法的示例程序:
```python
import numpy as np
import matplotlib.pyplot as plt
def kmeans(X, K, max_iters):
"""
K均值聚类算法
X: 输入数据矩阵,每一行表示一个样本
K: 簇的个数
max_iters: 最大迭代次数
"""
# 随机初始化质心
centroids = X[np.random.choice(X.shape[0], K, replace=False), :]
for i in range(max_iters):
# 计算每个样本到质心的距离
distances = np.sqrt(((X - centroids[:, np.newaxis])**2).sum(axis=2))
# 分配样本到最近的质心所在的簇中
labels = np.argmin(distances, axis=0)
# 更新质心
for j in range(K):
centroids[j] = X[labels == j, :].mean(axis=0)
return labels, centroids
# 生成数据
np.random.seed(1)
X1 = np.random.randn(50, 2) + np.array([5, 5])
X2 = np.random.randn(50, 2) + np.array([-5, -5])
X3 = np.random.randn(50, 2) + np.array([5, -5])
X = np.vstack([X1, X2, X3])
# 调用K均值聚类算法
K = 3
max_iters = 100
labels, centroids = kmeans(X, K, max_iters)
# 绘制结果
colors = ['r', 'g', 'b']
for i in range(K):
plt.scatter(X[labels == i, 0], X[labels == i, 1], c=colors[i], label='Cluster {}'.format(i+1))
plt.scatter(centroids[:, 0], centroids[:, 1], marker='x', s=200, linewidths=3, c='k', label='Centroids')
plt.legend()
plt.show()
```
该程序使用了numpy和matplotlib库。首先,程序随机初始化质心,并对数据进行最大迭代次数次迭代,计算每个样本到质心的距离,将每个样本分配到最近的质心所在的簇中,然后更新质心。最后,程序绘制聚类结果,其中不同簇的样本用不同颜色表示,质心用黑色叉号标注。
阅读全文
相关推荐
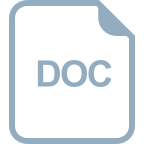
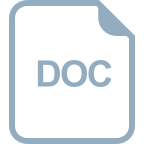
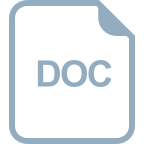







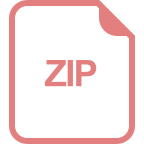
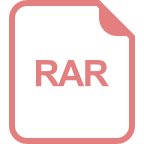






