用C++编写一个解决八数码问题的代码
时间: 2023-09-11 07:10:08 浏览: 164
好的,八数码问题是一种经典的搜索问题,可以用广度优先搜索(BFS)或者启发式搜索算法(如A*算法)来解决。下面是使用A*算法(估价函数为曼哈顿距离)的C++代码示例:
```c++
#include <iostream>
#include <cstdio>
#include <cstring>
#include <queue>
#include <algorithm>
using namespace std;
const int N = 3;
const int M = 1e5 + 5;
struct node
{
int g, h;
int a[N][N];
int x, y; // 空格的坐标
int pre; // 记录上一步的状态,输出解路径用
bool operator < (const node &t) const
{
return g + h > t.g + t.h;
}
}st, ed, u, v;
int dx[4] = {-1, 0, 1, 0}, dy[4] = {0, 1, 0, -1};
int f[M], pre[M]; // f[i]表示状态i在队列中的位置
int encode(node &t)
{
int res = 0;
for (int i = 0; i < N; i ++ )
for (int j = 0; j < N; j ++ )
res = res * 10 + t.a[i][j];
return res;
}
void decode(int code, node &t)
{
for (int i = N - 1; i >= 0; i -- )
for (int j = N - 1; j >= 0; j -- )
{
t.a[i][j] = code % 10;
code /= 10;
if (!t.a[i][j]) t.x = i, t.y = j;
}
}
int h(node &a, node &b)
{
int res = 0;
for (int i = 0; i < N; i ++ )
for (int j = 0; j < N; j ++ )
if (a.a[i][j])
{
int x = (a.a[i][j] - 1) / N, y = (a.a[i][j] - 1) % N;
res += abs(x - i) + abs(y - j);
}
return res;
}
void print(int u)
{
if (pre[u] != -1) print(pre[u]);
printf("%d\n", f[u]);
}
int main()
{
memset(f, -1, sizeof f);
memset(pre, -1, sizeof pre);
for (int i = 0; i < N; i ++ )
for (int j = 0; j < N; j ++ )
{
st.a[i][j] = i * N + j + 1;
ed.a[i][j] = (i + j + 1) % 9;
}
ed.a[N - 1][N - 1] = 0;
priority_queue<node> heap;
st.g = 0, st.h = h(st, ed);
f[encode(st)] = 0, heap.push(st);
while (heap.size())
{
u = heap.top(); heap.pop();
int code = encode(u);
if (code == encode(ed))
{
print(code);
break;
}
for (int i = 0; i < 4; i ++ )
{
int a = u.x + dx[i], b = u.y + dy[i];
if (a < 0 || a >= N || b < 0 || b >= N) continue;
memcpy(&v, &u, sizeof v);
swap(v.a[u.x][u.y], v.a[a][b]);
int code = encode(v);
if (f[code] == -1 || f[code] > f[encode(u)] + 1)
{
pre[code] = encode(u);
f[code] = f[encode(u)] + 1;
v.g = f[code], v.h = h(v, ed);
heap.push(v);
}
}
}
return 0;
}
```
这段代码使用A*算法求解八数码问题,其中的`encode`函数和`decode`函数用于将状态转化为整数以及将整数还原为状态,`h`函数用于计算估价函数(曼哈顿距离),`print`函数用于输出解路径。
阅读全文
相关推荐
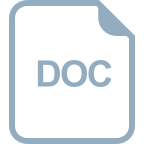
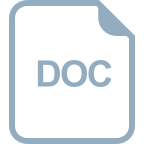
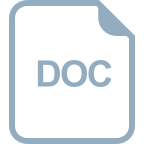
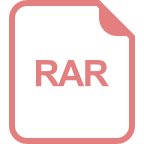


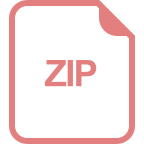
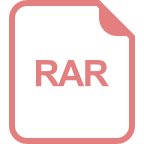
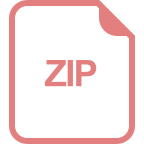
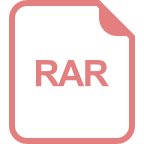
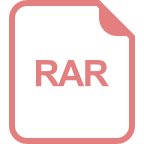
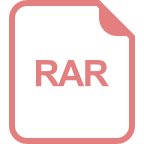
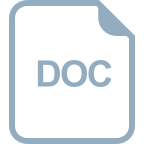





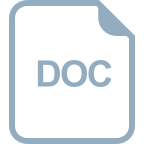