用c语言写出符合以下要求的代码【问题描述】假设一个算术表达式的所有操作数都是10以内的整数,请将该表达式转换为后缀表达式。 【输入形式】第一行输入一个中缀表达式字符串 【输出形式】第二行输出后缀表达式 【样例输入】43/(2-5)(2+3) 【样例输出】4325-/23+
时间: 2023-12-04 15:05:48 浏览: 97
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#define MAX_EXPR_LEN 100
#define MAX_STACK_SIZE 100
typedef struct {
int top;
char data[MAX_STACK_SIZE];
} Stack;
void initStack(Stack *stack) {
stack->top = -1;
}
int isEmpty(Stack *stack) {
return stack->top == -1;
}
int isFull(Stack *stack) {
return stack->top == MAX_STACK_SIZE - 1;
}
void push(Stack *stack, char c) {
if (isFull(stack)) {
printf("stack is full\n");
return;
}
stack->data[++stack->top] = c;
}
char pop(Stack *stack) {
if (isEmpty(stack)) {
printf("stack is empty\n");
return '\0';
}
return stack->data[stack->top--];
}
char top(Stack *stack) {
if (isEmpty(stack)) {
printf("stack is empty\n");
return '\0';
}
return stack->data[stack->top];
}
int isDigit(char c) {
return c >= '0' && c <= '9';
}
int priority(char op) {
switch (op) {
case '(':
return 1;
case '+':
case '-':
return 2;
case '*':
case '/':
return 3;
default:
return 0;
}
}
void infixToPostfix(char *expr, char *postfix) {
Stack stack;
initStack(&stack);
int len = strlen(expr);
int i, j;
char c;
for (i = 0, j = 0; i < len; i++) {
c = expr[i];
if (isDigit(c)) {
postfix[j++] = c;
} else if (c == '(') {
push(&stack, c);
} else if (c == ')') {
while (top(&stack) != '(') {
postfix[j++] = pop(&stack);
}
pop(&stack);
} else {
while (!isEmpty(&stack) && priority(c) <= priority(top(&stack))) {
postfix[j++] = pop(&stack);
}
push(&stack, c);
}
}
while (!isEmpty(&stack)) {
postfix[j++] = pop(&stack);
}
postfix[j] = '\0';
}
int main() {
char infix[MAX_EXPR_LEN], postfix[MAX_EXPR_LEN];
scanf("%s", infix);
infixToPostfix(infix, postfix);
printf("%s\n", postfix);
return 0;
}
阅读全文
相关推荐


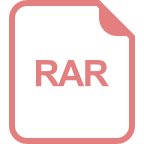















