stm32和esp8266串口通信
时间: 2023-09-02 15:12:06 浏览: 58
在STM32和ESP8266之间进行串口通信,需要将它们的串口连接起来,然后使用相应的代码进行数据传输。下面是一个简单的示例代码:
在STM32的代码中:
```c
#include "stm32f10x.h"
#include "stm32f10x_usart.h"
void USART1_Init(void)
{
GPIO_InitTypeDef GPIO_InitStructure;
USART_InitTypeDef USART_InitStructure;
RCC_APB2PeriphClockCmd(RCC_APB2Periph_USART1 | RCC_APB2Periph_GPIOA, ENABLE);
// USART1_TX -> PA9, USART1_RX -< PA10
GPIO_InitStructure.GPIO_Pin = GPIO_Pin_9;
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_AF_PP;
GPIO_InitStructure.GPIO_Speed = GPIO_Speed_50MHz;
GPIO_Init(GPIOA, &GPIO_InitStructure);
GPIO_InitStructure.GPIO_Pin = GPIO_Pin_10;
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_IN_FLOATING;
GPIO_InitStructure.GPIO_Speed = GPIO_Speed_50MHz;
GPIO_Init(GPIOA, &GPIO_InitStructure);
USART_InitStructure.USART_BaudRate = 115200;
USART_InitStructure.USART_WordLength = USART_WordLength_8b;
USART_InitStructure.USART_StopBits = USART_StopBits_1;
USART_InitStructure.USART_Parity = USART_Parity_No;
USART_InitStructure.USART_HardwareFlowControl = USART_HardwareFlowControl_None;
USART_InitStructure.USART_Mode = USART_Mode_Tx | USART_Mode_Rx;
USART_Init(USART1, &USART_InitStructure);
USART_Cmd(USART1, ENABLE);
}
void USART1_SendByte(uint8_t byte)
{
while (USART_GetFlagStatus(USART1, USART_FLAG_TXE) == RESET);
USART_SendData(USART1, byte);
}
uint8_t USART1_ReadByte(void)
{
while (USART_GetFlagStatus(USART1, USART_FLAG_RXNE) == RESET);
return USART_ReceiveData(USART1);
}
```
在ESP8266的代码中:
```c
#include <SoftwareSerial.h>
SoftwareSerial ESPserial(2, 3); // RX | TX
void setup()
{
Serial.begin(115200);
ESPserial.begin(115200);
}
void loop()
{
if (ESPserial.available())
Serial.write(ESPserial.read());
if (Serial.available())
ESPserial.write(Serial.read());
}
```
在上面的代码中,我们使用了STM32的USART1和ESP8266的软串口进行通信。在STM32的代码中,我们使用了USART1的TX和RX引脚连接到ESP8266的软串口上。在ESP8266的代码中,我们使用了SoftwareSerial库来实现软串口,并将其连接到STM32的USART1上。在loop()函数中,我们不断地检查两个串口的可用性,如果有数据可读,则将其写入对方的串口。
需要注意的是,以上代码只是一个示例,实际应用中需要根据具体的应用场景进行调整。
相关推荐
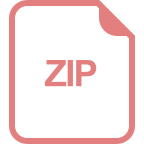
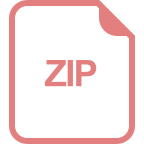
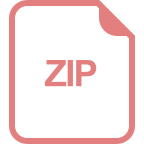














