stm32与esp8266串口2通信的代码
时间: 2024-01-01 15:03:24 浏览: 31
下面是一个简单的示例代码,演示了如何在STM32和ESP8266之间使用USART2进行串口通信。在这个示例中,STM32发送一个字符串给ESP8266,然后等待ESP8266的响应。
```
#include "stm32f10x.h"
#define USART2_TX_PIN GPIO_Pin_2
#define USART2_RX_PIN GPIO_Pin_3
#define USART2_GPIO GPIOA
USART_InitTypeDef USART_InitStructure;
void USART2_Init(void)
{
GPIO_InitTypeDef GPIO_InitStructure;
RCC_APB2PeriphClockCmd(RCC_APB2Periph_GPIOA | RCC_APB2Periph_AFIO | RCC_APB2Periph_USART1, ENABLE);
// Configure USART2 Tx (PA.2) as alternate function push-pull
GPIO_InitStructure.GPIO_Pin = USART2_TX_PIN;
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_AF_PP;
GPIO_InitStructure.GPIO_Speed = GPIO_Speed_50MHz;
GPIO_Init(USART2_GPIO, &GPIO_InitStructure);
// Configure USART2 Rx (PA.3) as input floating
GPIO_InitStructure.GPIO_Pin = USART2_RX_PIN;
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_IN_FLOATING;
GPIO_Init(USART2_GPIO, &GPIO_InitStructure);
// Configure USART2
USART_InitStructure.USART_BaudRate = 115200;
USART_InitStructure.USART_WordLength = USART_WordLength_8b;
USART_InitStructure.USART_StopBits = USART_StopBits_1;
USART_InitStructure.USART_Parity = USART_Parity_No;
USART_InitStructure.USART_HardwareFlowControl = USART_HardwareFlowControl_None;
USART_InitStructure.USART_Mode = USART_Mode_Tx | USART_Mode_Rx;
USART_Init(USART2, &USART_InitStructure);
// Enable USART2
USART_Cmd(USART2, ENABLE);
}
void USART2_SendString(char* s)
{
while(*s)
{
// Wait until data register is empty
while(!(USART2->SR & USART_SR_TXE));
USART_SendData(USART2, *s++);
}
}
int main(void)
{
char* message = "Hello, ESP8266!";
USART2_Init();
// Send message to ESP8266
USART2_SendString(message);
// Wait for response from ESP8266
while(!(USART2->SR & USART_SR_RXNE));
char response = USART_ReceiveData(USART2);
// Do something with the response...
while(1);
return 0;
}
```
请注意,这只是一个简单的示例代码,实际实现中可能需要考虑更多的错误检测和容错处理。同时,ESP8266的串口通信也需要相应的配置和代码来处理STM32发送的数据和发送回来的响应。
相关推荐
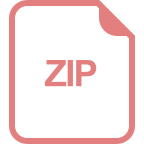
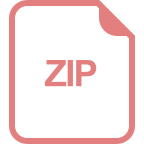
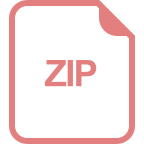
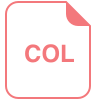
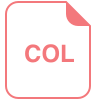
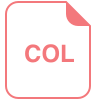
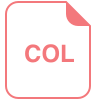
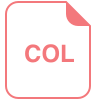









