后缀表达式+-*/优先级
时间: 2023-10-26 09:07:12 浏览: 80
后缀表达式是一种不含括号的表达式,其中运算符在操作数之后。在后缀表达式中,每个运算符的优先级是固定的,即乘除法优先于加减法。具体来说,当遇到一个运算符时,先将栈顶元素出栈作为右操作数,再将栈顶元素出栈作为左操作数,进行运算后将结果入栈。因此,后缀表达式的计算顺序是从左到右,符合人类的思维习惯,同时也方便计算机进行计算。
相关问题
8、表达式“S=A+B*(C-D)/E”的后缀表示形式可以为( ) A、XAB+CDE/-*= B、XA+BC-DE/*= C、XABCDE+*/= D、XABCD-*E/+=
选 B、XA+BC-DE/*=。
后缀表达式也叫逆波兰表达式,是一种不含括号的运算符表达式。将中缀表达式转换为后缀表达式的方法是使用栈来保存运算符,遍历中缀表达式,遇到操作数直接输出,遇到运算符则将栈中优先级大于或等于该运算符的运算符全部弹出并输出,再将该运算符入栈。最后将栈中剩余的运算符全部弹出并输出。
将中缀表达式“S=A+B*(C-D)/E”转换为后缀表达式的过程如下:
| 中缀表达式 | 栈 | 后缀表达式 |
|------------|-------------|-------------|
| | | X |
| A | | XA |
| + | + | XA |
| B | + | XAB |
| * | +* | XAB |
| ( | +*( | XAB |
| C | +*( | XABC |
| - | +-*( | XABC |
| D | +-*( | XABCD |
| ) | +* | XABC- |
| / | +/ | XABC- |
| E | +/ | XABC-E |
| | | XA+BC-DE/*= |
因此,后缀表达式为 XA+BC-DE/*=。
表达式a*b+c)-d的后缀表达式是( A. abcd*+- B. abc+*d- C. abc*+d- D.-+*abcd
表达式 "a * b + c) - d" 转换为后缀表达式(也称为逆波兰表示法 RPN),遵循操作符优先级和从左到右的顺序。后缀表达式中,操作数在前,操作符在后,并且括号内的表达式会先处理。
根据运算顺序,首先处理括号内的部分,然后是乘法和除法(从左到右),接着是加法和减法(同样从左到右)。所以表达式应为:
1. 先去掉括号,得到 "a * b + c - d"
2. 将乘法和减法移到操作数之后:a b * + c - d
3. 将乘法和加法转换为后缀:a b * + c - d (因为它们的优先级相同,从左到右)
因此,正确答案是 B.
相关推荐
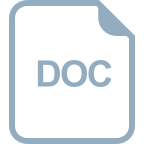
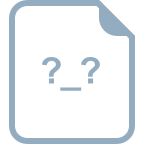
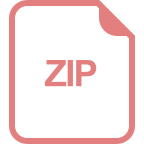












