栈中缀表达式转后缀c++
时间: 2023-08-18 20:05:00 浏览: 49
将中缀表达式转换为后缀表达式的算法可以使用栈来实现。下面是一个使用 C++ 实现的示例代码:
```cpp
#include <iostream>
#include <stack>
#include <string>
// 判断运算符的优先级
int getPriority(char op) {
if (op == '+' || op == '-') {
return 1;
} else if (op == '*' || op == '/') {
return 2;
}
return 0;
}
// 将中缀表达式转换为后缀表达式
std::string infixToPostfix(std::string infix) {
std::stack<char> opStack;
std::string postfix;
for (char ch : infix) {
if (isalnum(ch)) { // 如果是字母或数字,直接加入后缀表达式
postfix += ch; } else if (ch == '(') { // 如果是左括号,入栈
opStack.push(ch);
} else if (ch == ')') { // 如果是右括号,将栈中的运算符弹出,加入后缀表达式,直到遇到左括号
while (!opStack.empty() && opStack.top() != '(') {
postfix += opStack.top();
opStack.pop();
}
opStack.pop(); // 弹出左括号
} else { // 如果是运算符
while (!opStack.empty() && opStack.top() != '(' && getPriority(ch) <= getPriority(opStack.top())) {
postfix += opStack.top();
opStack.pop();
}
opStack.push(ch);
}
}
while (!opStack.empty()) { // 将栈中剩余的运算符加入后缀表达式
postfix += opStack.top();
opStack.pop();
}
return postfix;
}
int main() {
std::string infixExpression;
std::cout << "Enter an infix expression: ";
std::getline(std::cin, infixExpression);
std::string postfixExpression = infixToPostfix(infixExpression);
std::cout << "Postfix expression: " << postfixExpression << std::endl;
return 0;
}
```
你可以使用 `std::getline()` 函数输入中缀表达式,然后调用 `infixToPostfix()` 函数将其转换为后缀表达式。最后,将转换后的后缀表达式打印出来。
请注意,该示例代码仅处理四则运算符和括号,如果需要处理其他运算符或函数,你需要根据具体的需求进行修改。
相关推荐
















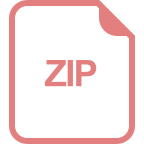
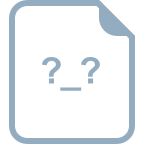