1用两种方法绘制z=2x2-3y²的图形,并添加标题和坐标轴标签、
时间: 2024-10-28 17:11:46 浏览: 31
要绘制函数 \( z = 2x^2 - 3y^2 \) 的三维图形,你可以选择两种常见的绘图方法:
**方法一:Python Matplotlib**
如果你熟悉Python和Matplotlib库,可以这样做:
```python
import numpy as np
import matplotlib.pyplot as plt
from mpl_toolkits.mplot3d import Axes3D
# 定义数据范围
x = np.linspace(-5, 5, 100)
y = np.linspace(-5, 5, 100)
# 创建网格
X, Y = np.meshgrid(x, y)
Z = 2 * X**2 - 3 * Y**2
# 绘制三维图形
fig = plt.figure()
ax = fig.add_subplot(111, projection='3d')
ax.plot_surface(X, Y, Z, cmap='viridis', edgecolor='none')
# 添加标题和坐标轴标签
ax.set_title('三维函数 z=2x^2-3y^2')
ax.set_xlabel('X轴')
ax.set_ylabel('Y轴')
ax.set_zlabel('Z轴')
plt.show()
```
**方法二:JavaScript (Three.js)**
对于Web应用,可以使用JavaScript的Three.js库创建交互式3D图形:
```html
<!DOCTYPE html>
<html lang="en">
<head>
<script src="https://cdn.jsdelivr.net/npm/three@0.132.2/build/three.min.js"></script>
<!-- ...其他依赖库... -->
</head>
<body>
<script>
// 初始化场景
const scene = new THREE.Scene();
const camera = new THREE.PerspectiveCamera(75, window.innerWidth / window.innerHeight, 0.1, 1000);
// 创建网格几何体
const geometry = new THREE.BoxGeometry(5, 5, 5);
const material = new THREE.MeshBasicMaterial({ color: 'blue' });
const surface = new THREE.Mesh(geometry, material);
surface.rotation.x = -Math.PI / 2; // 使得y轴朝上
scene.add(surface);
// 更新渲染
function animate() {
requestAnimationFrame(animate);
surface.position.set(x, y, z); // 用X, Y, Z替换计算得到的实际值
renderer.render(scene, camera);
}
// ...在HTML中添加webGL渲染器...
// 加入标题和坐标轴标签
// (需要手动设置,例如通过CSS或DOM操作)
animate();
</script>
</body>
</html>
```
在这两个例子中,你需要用数值代替`x`, `y`, 和 `z` 来计算实际的点并绘制曲面。记住在每个环境中调整合适的图形显示设置。
阅读全文
相关推荐
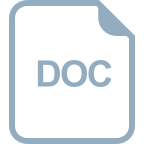
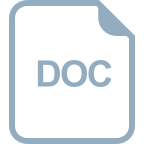
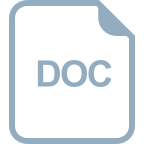



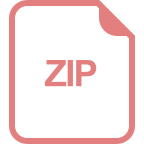
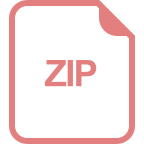
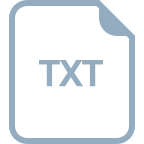
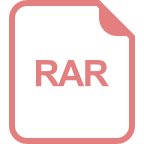
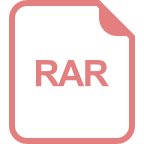
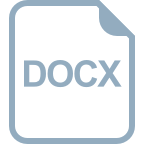
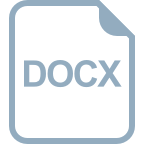
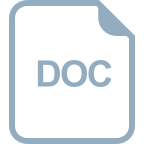
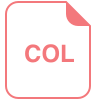



