im_gaussian_h = np.zeros((len(img) - 2, len(img[0]) - 2))
时间: 2024-05-29 22:12:14 浏览: 5
This line of code creates a 2D array of zeros with dimensions of (len(img) - 2, len(img[0]) - 2). The purpose of this array is likely to store the results of convolving the image with a Gaussian filter, which is a common image processing technique for smoothing and reducing noise. The resulting array will have slightly smaller dimensions than the original image because the edges of the image cannot be fully convolved with the filter.
相关问题
import cv2 import numpy as np def retinex(img, sigma_list): retinex = np.zeros_like(img) for sigma in sigma_list: img_blur = cv2.GaussianBlur(img, (0, 0), sigma) img_blur = np.where(img_blur == 0, 0.1, img_blur) retinex += np.log10(img) - np.log10(img_blur) retinex = retinex / len(sigma_list) retinex = np.where(retinex < 0, 0, retinex) retinex = np.where(retinex > 1, 1, retinex) return retinex def enhance_image(img): hsi = cv2.cvtColor(img, cv2.COLOR_BGR2HSV) h, s, i = cv2.split(hsi) i_enhanced = retinex(i.astype(np.float64), [15, 80, 250]) i_enhanced = (i_enhanced * 255).astype(np.uint8) s_enhanced = cv2.equalizeHist(s) hsi_enhanced = cv2.merge([h, s_enhanced, i_enhanced]) enhanced_img = cv2.cvtColor(hsi_enhanced, cv2.COLOR_HSV2BGR) return enhanced_img img = cv2.imread('low_illumination_1.png') enhanced_img = enhance_image(img) cv2.imshow('Enhanced Image', enhanced_img) cv2.waitKey(0) cv2.destroyAllWindows()
这是一段基于Retinex算法对图像进行增强的Python代码。Retinex算法是一种用于图像增强的经典算法,它可以增强图像的对比度和颜色鲜艳度,同时可以保留图像的细节信息。该算法的基本思想是将图像分解为多个尺度的高斯模糊图像,然后通过计算不同尺度的高斯模糊图像与原始图像之间的差异来获得图像的反射性和色彩性信息。在这段代码中,首先将输入图像转换为HSI颜色空间,然后对亮度通道进行Retinex增强处理,对饱和度通道进行直方图均衡化处理,最后将三个通道重新组合成BGR颜色空间的图像输出。
def multiScaleRetinex(img, sigma_list): retinex = np.zeros_like(img) for sigma in sigma_list: retinex += singleScaleRetinex(img, sigma) retinex = retinex / len(sigma_list) return retinex
这是一个多尺度Retinex算法的Python代码实现。Retinex算法是一种图像增强算法,它试图在保留图像结构的同时消除光照影响。
这个函数的输入是一张图像和一个sigma值的列表,sigma值表示高斯滤波器的标准差,用于在不同尺度下对图像进行滤波。接着,该函数调用singleScaleRetinex函数对每个sigma值进行处理,并将结果累加到一个输出图像中。最后,将输出图像除以sigma值的个数,得到最终的多尺度Retinex增强图像。
以下是singleScaleRetinex函数的代码实现,用于对单个sigma值对图像进行处理:
```
def singleScaleRetinex(img, sigma):
blur = cv2.GaussianBlur(img, (0, 0), sigma)
retinex = np.log10(img) - np.log10(blur)
return retinex
```
该函数首先对图像进行高斯滤波,然后计算原始图像与滤波后的图像的对数差,得到一个Retinex增强图像。
相关推荐
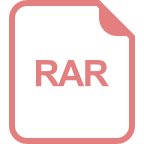
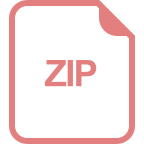











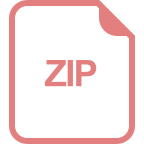
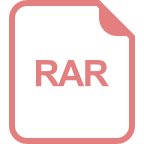