调用代价敏感损失函数代码示例
时间: 2023-09-30 21:02:56 浏览: 53
以下是使用Python编写的代价敏感损失函数的示例代码:
```python
import tensorflow as tf
def cost_sensitive_loss(y_true, y_pred):
# 定义代价矩阵
cost_matrix = tf.constant([[0.0, 1.0], [5.0, 0.0]])
# 计算代价敏感损失
loss = tf.reduce_mean(tf.multiply(cost_matrix[:, 0], tf.multiply(1 - y_true, y_pred)) +
tf.multiply(cost_matrix[:, 1], tf.multiply(y_true, 1 - y_pred)))
return loss
```
在上面的代码中,我们首先定义了一个代价矩阵,其中第一行表示将正类预测为负类的代价,第二行表示将负类预测为正类的代价。然后,我们使用TensorFlow的reduce_mean函数计算了代价敏感损失。具体来说,我们分别计算了将正类预测为负类和将负类预测为正类的代价,然后将它们加起来并取平均值作为最终的损失。
请注意,此函数假定y_true和y_pred都是形状为(batch_size, num_classes)的张量,其中每一行表示一个样本的类别标签和预测结果。如果你的情况不同,你需要相应地修改函数的实现。
相关问题
如何修改matlab A*算法代价函数
在Matlab中,可以通过修改代价函数来改变A*算法的行为。代价函数是一个计算从起点到当前节点的代价的函数。具体来说,它将当前节点与起点之间的距离加上当前节点的启发式估计值,以计算当前节点的总代价。启发式估计值是从当前节点到目标节点的估计距离,通常使用曼哈顿距离或欧几里得距离来计算。
以下是一个简单的A*算法的代码示例,其中代价函数被定义为距离加上启发式估计值:
```matlab
function [path, cost] = astar(startNode, goalNode, adjMatrix, heuristic)
% Initialize the algorithm
visited = false(size(adjMatrix, 1), 1);
gScore = Inf(size(adjMatrix, 1), 1);
fScore = Inf(size(adjMatrix, 1), 1);
gScore(startNode) = 0;
fScore(startNode) = heuristic(startNode, goalNode);
% Search for the goal node
while ~all(visited)
% Find the node with the lowest fScore
[~, current] = min(fScore(~visited));
visited(current) = true;
% Check if we've reached the goal node
if current == goalNode
path = backtrackPath(goalNode);
cost = gScore(goalNode);
return
end
% Update the gScore and fScore of neighbors
neighbors = find(adjMatrix(current, :));
for i = 1:length(neighbors)
neighbor = neighbors(i);
if visited(neighbor)
continue
end
tentativeGScore = gScore(current) + adjMatrix(current, neighbor);
if tentativeGScore < gScore(neighbor)
gScore(neighbor) = tentativeGScore;
fScore(neighbor) = gScore(neighbor) + heuristic(neighbor, goalNode);
end
end
end
% If we get here, there's no path to the goal node
path = [];
cost = Inf;
end
function path = backtrackPath(node)
% Backtrack from the goal node to the start node
path = node;
while ~isempty(node.parent)
node = node.parent;
path = [node path];
end
end
```
如果您想修改代价函数,只需更改启发式估计值的计算方式即可。例如,如果您想使用曼哈顿距离作为启发式估计值,可以将启发式函数定义为:
```matlab
function h = manhattanDistance(node, goalNode)
% Compute the Manhattan distance between node and goalNode
dx = abs(node.x - goalNode.x);
dy = abs(node.y - goalNode.y);
h = dx + dy;
end
```
然后,在调用A*算法时,将启发式函数作为参数传递:
```matlab
path = astar(startNode, goalNode, adjMatrix, @manhattanDistance);
```
您可以根据需要修改代价函数,以适应不同的问题和场景。
在不调用函数的情况下实现Robust Fuzzy C-Means (RFCM)
要在不调用函数的情况下实现Robust Fuzzy C-Means (RFCM),你需要按照RFCM算法的步骤进行手动编码实现。以下是RFCM算法的主要步骤:
1. 准备数据:将数据集准备成一个矩阵,每一行代表一个样本,每一列代表一个特征。
2. 初始化隶属度矩阵:随机生成一个与数据集大小相同的隶属度矩阵U,其中每个元素表示样本对于每个聚类的隶属度。
3. 迭代更新隶属度和聚类中心:根据当前的隶属度矩阵U,计算每个样本对于每个聚类的隶属度,并更新聚类中心。
4. 计算鲁棒代价函数:根据更新后的聚类中心,计算每个样本到聚类中心的距离,并基于鲁棒代价函数进行修正。
5. 判断停止条件:根据设定的停止条件(如最大迭代次数或者聚类中心的变化率),判断是否继续迭代。
以下是一个简单的RFCM算法的代码示例:
```matlab
function [U, centroids] = rfcm(data, num_clusters, max_iter)
[num_samples, num_features] = size(data);
U = rand(num_samples, num_clusters); % 随机初始化隶属度矩阵
U = U ./ sum(U, 2);
centroids = rand(num_clusters, num_features); % 随机初始化聚类中心
for i = 1:max_iter
% 更新聚类中心
centroids = (U' * data) ./ sum(U)';
% 更新隶属度矩阵
distances = pdist2(data, centroids);
U = 1 ./ ((distances ./ distances).^2);
U = U ./ sum(U, 2);
% 计算鲁棒代价函数
e = abs(data - centroids(cluster_labels, :));
e_median = median(e(:));
rho = 1.4826 * e_median;
w = 1 ./ (1 + (e / (3 * rho)).^2);
% 更新隶属度矩阵
U = U .* (w .^ 2);
U = U ./ sum(U, 2);
end
[~, cluster_labels] = max(U, [], 2);
end
```
在这个示例中,`data`是数据集,`num_clusters`是聚类簇的数量,`max_iter`是最大迭代次数。函数返回最终的隶属度矩阵U和聚类中心centroids。
请注意,这只是一个简单的RFCM算法实现示例,实际应用中可能需要根据具体需求进行一些调整和优化。同时,这里使用了`pdist2`函数来计算样本间的距离,如果不想使用该函数,也可以手动计算距离。
相关推荐
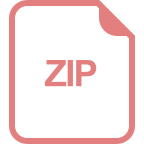
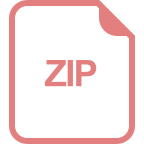
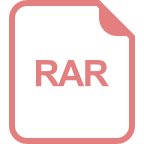












