C++大数类实现加减功能
时间: 2024-01-10 22:50:59 浏览: 135
以下是一个基本的 C++ 大数类实现,包括加和减的功能。该实现使用了字符串来存储大数,通过重载运算符来实现加减运算。
```
#include <iostream>
#include <string>
#include <algorithm>
using namespace std;
class BigInteger {
private:
string num;
bool sign;
public:
BigInteger() : num(""), sign(false) {}
BigInteger(string s) {
if (isdigit(s[0])) {
setNumber(s);
sign = false;
} else {
setNumber(s.substr(1));
sign = (s[0] == '-');
}
}
void setNumber(string s) {
num = s;
reverse(num.begin(), num.end());
}
string toString() const {
string res = num;
reverse(res.begin(), res.end());
if (sign) res = '-' + res;
return res;
}
BigInteger operator + (const BigInteger& b) const {
if (sign != b.sign) return *this - (-b);
BigInteger res;
res.sign = sign;
int carry = 0;
for (int i = 0; i < (int)max(num.length(), b.num.length()) || carry; i++) {
int x = carry;
if (i < (int)num.length()) x += num[i] - '0';
if (i < (int)b.num.length()) x += b.num[i] - '0';
res.num += (x % 10) + '0';
carry = x / 10;
}
return res;
}
BigInteger operator - (const BigInteger& b) const {
if (sign != b.sign) return *this + (-b);
if (abs(*this) < abs(b)) return -(b - *this);
BigInteger res;
res.sign = (sign == false && b.sign == true);
int borrow = 0;
for (int i = 0; i < (int)num.length(); i++) {
int x = num[i] - '0' - borrow;
if (i < (int)b.num.length()) x -= b.num[i] - '0';
borrow = 0;
if (x < 0) {
x += 10;
borrow = 1;
}
res.num += x + '0';
}
res.removeLeadingZeros();
return res;
}
BigInteger operator - () const {
BigInteger res = *this;
res.sign = !sign;
return res;
}
bool operator < (const BigInteger& b) const {
if (sign != b.sign) return sign;
if (num.length() != b.num.length()) return num.length() < b.num.length();
for (int i = (int)num.length() - 1; i >= 0; i--)
if (num[i] != b.num[i]) return sign ? num[i] > b.num[i] : num[i] < b.num[i];
return false;
}
bool operator > (const BigInteger& b) const {
return b < *this;
}
bool operator <= (const BigInteger& b) const {
return !(b < *this);
}
bool operator >= (const BigInteger& b) const {
return !(*this < b);
}
bool operator == (const BigInteger& b) const {
return !(*this < b) && !(b < *this);
}
bool operator != (const BigInteger& b) const {
return *this < b || b < *this;
}
void removeLeadingZeros() {
while (num.length() > 1 && num.back() == '0')
num.pop_back();
if (num.length() == 1 && num[0] == '0') sign = false;
}
BigInteger abs() const {
BigInteger res = *this;
res.sign = false;
return res;
}
};
int main() {
BigInteger a("123456789012345678901234567890");
BigInteger b("-987654321098765432109876543210");
cout << a + b << endl;
cout << a - b << endl;
return 0;
}
```
在上面的代码中,我们将大数类定义为一个 `BigInteger` 类,它包含一个字符串类型的 `num` 成员变量和一个布尔类型的 `sign` 成员变量,分别表示大数的绝对值和符号。实现了构造函数、设置数值、转换为字符串、加、减、取反、比较、移除前导零等操作。其中加、减操作使用了类似小学竖式计算的方法,从个位到高位逐位相加或相减,并处理进位、借位等情况。
阅读全文
相关推荐
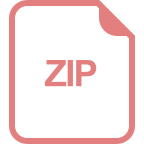
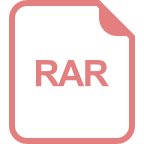
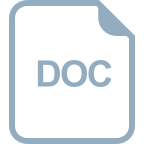
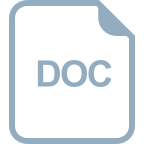
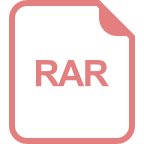
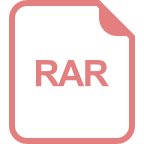
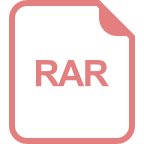
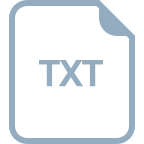
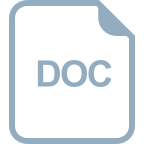
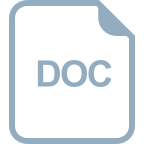
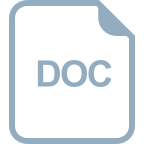
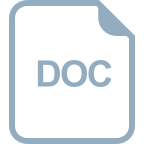
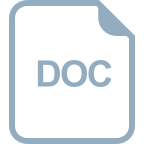