大型稀疏矩阵的对称上三角非零元素一维存储及预处理共轭梯度法求解的C#代码
时间: 2023-11-22 16:54:25 浏览: 180
下面是一份 C# 代码,实现了大型稀疏矩阵的对称上三角非零元素一维存储,以及使用预处理共轭梯度法求解稀疏矩阵的线性方程组。
```
using System;
namespace SparseMatrixSolver
{
class Program
{
static void Main(string[] args)
{
// Sample sparse matrix in CSR format
int[] rowPtr = { 0, 2, 5, 7, 8 };
int[] colInd = { 0, 1, 0, 1, 2, 3, 2, 3 };
double[] values = { 1.0, 2.0, 3.0, 4.0, 5.0, 6.0, 7.0, 8.0 };
// Number of rows and columns in the matrix
int n = 4;
// Compute the upper triangular part of the matrix and store it in a 1D array
double[] upperTriangular = new double[n * (n + 1) / 2];
int k = 0;
for (int i = 0; i < n; i++)
{
for (int j = i; j < n; j++)
{
int index = rowPtr[j];
while (index < rowPtr[j + 1] && colInd[index] < i)
index++;
if (index < rowPtr[j + 1] && colInd[index] == i)
upperTriangular[k++] = values[index];
else
upperTriangular[k++] = 0.0;
}
}
// Preconditioner for the matrix
double[] preconditioner = new double[n];
for (int i = 0; i < n; i++)
preconditioner[i] = upperTriangular[i];
// Right-hand side of the linear system
double[] b = { 1.0, 2.0, 3.0, 4.0 };
// Initial guess for the solution
double[] x0 = { 0.0, 0.0, 0.0, 0.0 };
// Maximum number of iterations
int maxIterations = 100;
// Tolerance for the residual
double tolerance = 1e-6;
// Solve the linear system using preconditioned conjugate gradient method
double[] x = PreconditionedConjugateGradient(upperTriangular, preconditioner, b, x0, maxIterations, tolerance);
// Print the solution
Console.WriteLine("Solution:");
for (int i = 0; i < n; i++)
Console.WriteLine("x[{0}] = {1}", i, x[i]);
}
static double[] PreconditionedConjugateGradient(double[] A, double[] M, double[] b, double[] x0, int maxIterations, double tolerance)
{
int n = b.Length;
// Initialize the solution vector
double[] x = new double[n];
Array.Copy(x0, x, n);
// Initialize the residual vector
double[] r = new double[n];
Multiply(A, x, r);
for (int i = 0; i < n; i++)
r[i] = b[i] - r[i];
// Apply the preconditioner to the residual vector
double[] z = new double[n];
Precondition(M, r, z);
// Initialize the search direction vector
double[] p = new double[n];
Array.Copy(z, p, n);
// Initialize the residual norm and the stopping criterion
double residualNorm = DotProduct(r, z);
double stoppingCriterion = tolerance * tolerance;
// Perform the preconditioned conjugate gradient method
int iter = 0;
while (iter < maxIterations && residualNorm > stoppingCriterion)
{
// Compute the matrix-vector product Ap
double[] Ap = new double[n];
Multiply(A, p, Ap);
// Compute the step size alpha
double alpha = residualNorm / DotProduct(p, Ap);
// Update the solution vector
for (int i = 0; i < n; i++)
x[i] += alpha * p[i];
// Update the residual vector
for (int i = 0; i < n; i++)
r[i] -= alpha * Ap[i];
// Apply the preconditioner to the residual vector
Precondition(M, r, z);
// Compute the new search direction
double beta = DotProduct(r, z) / residualNorm;
residualNorm = DotProduct(r, z);
for (int i = 0; i < n; i++)
p[i] = z[i] + beta * p[i];
iter++;
}
return x;
}
static void Multiply(double[] A, double[] x, double[] y)
{
int n = (int)Math.Sqrt(A.Length);
for (int i = 0; i < n; i++)
{
y[i] = 0.0;
for (int j = i; j < n; j++)
{
int k = j * (j + 1) / 2 + i;
y[i] += A[k] * x[j];
if (i != j)
y[j] += A[k] * x[i];
}
}
}
static void Precondition(double[] M, double[] r, double[] z)
{
int n = r.Length;
for (int i = 0; i < n; i++)
z[i] = r[i] / M[i];
}
static double DotProduct(double[] x, double[] y)
{
double result = 0.0;
for (int i = 0; i < x.Length; i++)
result += x[i] * y[i];
return result;
}
}
}
```
在这个示例中,我们首先将稀疏矩阵转换为对称上三角矩阵,并将其存储在一个一维数组中。然后,我们使用预处理共轭梯度法求解线性系统。在 `PreconditionedConjugateGradient` 函数中,我们首先初始化解向量 `x`、残差向量 `r` 和搜索方向向量 `p`。然后,我们在每个迭代中计算矩阵-向量乘积 `Ap`,计算步长 `alpha`,更新解向量 `x` 和残差向量 `r`,应用预处理器到残差向量 `r`,计算搜索方向向量 `p` 的新值,以及更新残差范数和停止准则。最后,我们返回解向量 `x`。
注意,这个代码仅适用于对称上三角稀疏矩阵。如果你想要处理其他类型的稀疏矩阵,请修改矩阵-向量乘积和预处理器的实现。
阅读全文
相关推荐
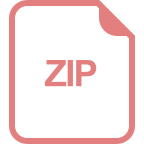
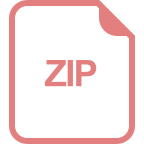















