def save(index, saveDict,modelDir='checkpoint',pthType='epoch'): if os.path.dirname(modelDir)!='' and not os.path.exists(os.path.dirname(modelDir)): os.makedirs(os.path.dirname(modelDir)) torch.save(saveDict, modelDir+'/encoder_{}_{:08d}.pth'.format(pthType, index))
时间: 2024-04-19 07:27:23 浏览: 6
这段代码定义了一个保存模型的函数`save()`。函数接受以下参数:
- `index`:表示当前的索引或者轮次。
- `saveDict`:表示要保存的模型字典或者状态。
- `modelDir`:表示保存模型的目录,默认为`checkpoint`。
- `pthType`:表示保存模型的类型,默认为`epoch`。
函数的功能是将模型保存到指定的目录下,并使用给定的索引和类型命名保存的文件。具体操作如下:
1. 首先,通过检查`modelDir`中指定的目录是否存在,如果不存在则创建该目录(如果`modelDir`中包含父目录,则也会创建父目录)。
2. 然后,使用`torch.save()`函数将`saveDict`保存为`.pth`文件到`modelDir`目录下。文件名的格式为`encoder_{pthType}_{index:08d}.pth`,其中`{pthType}`和`{index}`会根据参数的值进行替换。
这个函数的作用是方便保存训练过程中的模型,并且可以根据需要自定义保存的目录和文件名。
相关问题
save_dir = os.path.join(os.getcwd(), 'saved_models') filepath = "model_{epoch:02d}-{val_acc:.2f}.hdf5" checkpoint = ModelCheckpoint(os.path.join(save_dir, filepath), monitor='val_acc',verbose=1, save_best_only=True)
这段代码是用来设置模型检查点的。在训练深度学习模型时,我们通常会在每个 epoch 结束时评估模型在验证集上的表现。为了避免过拟合,我们可以在验证集上表现最好的模型进行保存。这就是所谓的“检查点”。
在这段代码中,我们首先定义了一个保存模型的目录 `save_dir`,它是当前工作目录下的 `saved_models` 目录。然后我们定义了一个文件名 `filepath`,它包含了两个变量 `epoch` 和 `val_acc`,分别表示当前模型所处的 epoch 数和在验证集上的准确率。这样每个 epoch 结束时,Keras 就会将模型保存到 `save_dir` 目录下,并且文件名会包含当前 epoch 数和验证集准确率。
最后,我们使用 `ModelCheckpoint` 该类来设置检查点。`monitor` 参数指定了需要监控的指标,这里是验证集准确率;`verbose` 参数指定了日志输出的详细程度,这里设置为 1 表示输出较详细;`save_best_only` 参数指定是否只保存在验证集上表现最好的模型。
def reload(checkpoint,modelDir='checkpoint',pthType='epoch',print=print,multiGPU=False): try: if checkpoint is not None: saveDict = torch.load(modelDir+'/encoder_{}_{:08d}.pth'.format(pthType, checkpoint),map_location=device) pth = modelDir+'/encoder_{}_{:08d}.pth'.format(pthType, checkpoint) if checkpoint is None: saveDict = torch.load(modelDir,map_location=device) pth = modelDir saveDict['path'] = pth # print('load: ',pth) if multiGPU: from collections import OrderedDict state_dict = OrderedDict() new_state_dict = OrderedDict() for k, v in saveDict['encoder'].items(): name = k[7:] # remove `module.` state_dict[name] = v saveDict['encoder'] = state_dict return saveDict except Exception as e: print('**warning**',e,' start from initial model') # saveDict['path'] = e return None
这段代码定义了一个重新加载模型的函数`reload()`。函数接受以下参数:
- `checkpoint`:表示要重新加载的模型的索引或者轮次。如果为`None`,则加载整个模型目录。
- `modelDir`:表示保存模型的目录,默认为`checkpoint`。
- `pthType`:表示保存模型的类型,默认为`epoch`。
- `print`:表示打印函数,默认为内置的`print()`函数。
- `multiGPU`:表示是否使用多个GPU,默认为`False`。
函数的功能是根据给定的参数重新加载模型。具体操作如下:
1. 首先,通过检查`checkpoint`参数,确定要重新加载的模型文件。
- 如果`checkpoint`不为`None`,则使用模型目录和给定的索引或轮次来构建模型文件的路径。
- 如果`checkpoint`为`None`,则直接使用模型目录作为模型文件的路径。
2. 然后,使用`torch.load()`函数加载模型文件,并将结果保存到`saveDict`中。
3. 接着,将模型文件的路径保存到`saveDict['path']`中。
4. 如果设置了`multiGPU`为`True`,则将加载的模型字典中的键名中的`module.`前缀去除,以适应多GPU训练时保存的模型字典的格式。
5. 最后,返回加载的模型字典。
这个函数的作用是方便重新加载已保存的模型,并且可以根据需要选择重新加载整个模型目录或者指定索引或轮次的模型文件。如果重新加载失败,函数会打印警告信息并返回`None`。
相关推荐
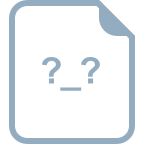












