友元函数如何應用在運算符重載?
时间: 2024-09-19 13:08:29 浏览: 91
友元函数是一种特殊的朋友(Friend)关系,在C++中,它允许非成员函数访问类的私有和保护成员,这对于实现运算符重载特别有用。当我们需要自定义某个运算符的行为,比如`+`, `-`, `*`, `/`, `==`等,通常会在该运算符所在的类之外定义一个友元函数。
友元函数可以用来提供运算符的具体实现。例如,如果你有一个名为`Vector`的类,想要让它支持向量相加,你可以这样做:
```cpp
class Vector {
private:
// ...
public:
// 运算符+的声明
friend Vector operator+(const Vector& v1, const Vector& v2);
};
// 实现运算符+的友元函数
Vector operator+(const Vector& v1, const Vector& v2) {
// 计算两个向量的元素对应位置的和
// ...
return result;
}
```
在这里,`operator+`就是一个友元函数,它可以直接访问`Vector`类的私有成员,从而完成向量的加法操作。这样做的好处是可以保持运算符的语法一致性,同时避免了将复杂的计算放到类内。
相关问题
编写程序实现 Complex 类,包含两个整数成员变量实部和虚部。 .友元函数实现++运算符重载;.成员函数实现﹣﹣运算符重载;.友元函数实现*运算符重载;.实现输入和输出运算符重载;
好的,我来为您编写一个 Complex 类实现以下功能:
1. 包含两个整数成员变量实部和虚部;
2. 友元函数实现++运算符重载;
3. 成员函数实现﹣﹣运算符重载;
4. 友元函数实现*运算符重载;
5. 实现输入和输出运算符重载。
代码如下:
```c++
#include <iostream>
using namespace std;
class Complex {
private:
int real; // 实部
int imag; // 虚部
public:
// 构造函数
Complex(int real = 0, int imag = 0) {
this->real = real;
this->imag = imag;
}
// 友元函数实现++运算符重载
friend Complex operator++(Complex &a, int) {
Complex old = a;
a.real++;
a.imag++;
return old;
}
// 成员函数实现﹣﹣运算符重载
Complex operator--() {
real--;
imag--;
return *this;
}
// 友元函数实现*运算符重载
friend Complex operator*(const Complex &a, const Complex &b) {
Complex c;
c.real = a.real * b.real - a.imag * b.imag;
c.imag = a.real * b.imag + a.imag * b.real;
return c;
}
// 实现输入和输出运算符重载
friend ostream& operator<<(ostream &out, const Complex &c) {
out << c.real << "+" << c.imag << "i";
return out;
}
friend istream& operator>>(istream &in, Complex &c) {
cout << "请输入实部和虚部,格式为:实部 虚部" << endl;
in >> c.real >> c.imag;
return in;
}
};
int main() {
Complex c1, c2;
cin >> c1 >> c2;
cout << "c1 = " << c1 << endl;
cout << "c2 = " << c2 << endl;
Complex c3 = c1 * c2;
cout << "c1 * c2 = " << c3 << endl;
cout << "c1++ = " << c1++ << endl;
cout << "--c2 = " << --c2 << endl;
return 0;
}
```
您可以在 `main()` 函数中进行测试,可以自行修改输入输出的值。
友元函数的加号运算符重载
友元函数的加号运算符重载通常用于实现两个对象相加的操作。在类中定义加号运算符重载函数时,必须将该函数声明为友元函数,以便该函数能够直接访问该类的私有成员变量。友元函数的声明方式为:`friend 返回类型 operator+(const 类名& obj1, const 类名& obj2);`
其中,`obj1`和`obj2`代表两个要相加的对象,`返回类型`代表相加后的结果类型。在函数中,可以使用两个对象的私有成员变量进行计算,并返回计算结果。例如:
```
class MyClass {
private:
int x;
public:
MyClass(int val) : x(val) {}
friend MyClass operator+(const MyClass& obj1, const MyClass& obj2);
};
MyClass operator+(const MyClass& obj1, const MyClass& obj2) {
return MyClass(obj1.x + obj2.x);
}
```
在上述代码中,我们定义了一个名为`MyClass`的类,并在其中定义了一个名为`operator+`的友元函数,该函数用于将两个`MyClass`类型的对象相加。在函数中,我们使用两个对象的私有成员变量`x`进行计算,最终返回一个新的`MyClass`对象,该对象的`x`值为相加后的结果。
阅读全文
相关推荐
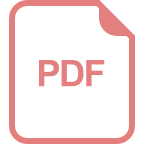
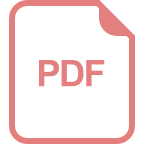
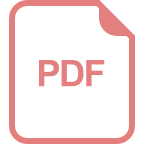
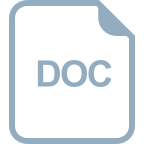
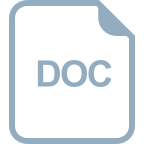
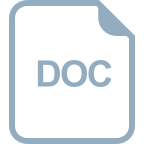






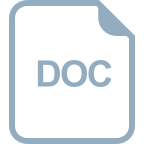



