c语言计算一元二次方程的根
时间: 2024-09-27 08:07:22 浏览: 50
在C语言中,可以使用数学库函数如`sqrt()`来计算一元二次方程ax² + bx + c = 0的根,其中a、b、c是常数。由于解二次方程需要使用到公式 `-b ± sqrt(b² - 4ac) / (2a)`,我们可以创建一个函数来处理这个问题。以下是一个简单的示例:
```c
#include <stdio.h>
#include <math.h>
// 函数声明,接受系数a, b, c
void quadraticRoots(double a, double b, double c, double* roots);
int main() {
// 示例输入系数
double a = 1.0, b = -3.0, c = 2.0;
// 声明存储两个根的空间
double root1, root2;
// 调用函数并获取结果
quadraticRoots(a, b, c, &root1);
quadraticRoots(a, b, c, &root2); // 如果有重复根,这里会覆盖之前的值
printf("The roots of the quadratic equation are %.2lf and %.2lf\n", root1, root2);
return 0;
}
// 计算二次方程的根
void quadraticRoots(double a, double b, double c, double* roots) {
double discriminant = b * b - 4 * a * c;
if (discriminant > 0) { // 有两个不相等的实根
*roots = (-b + sqrt(discriminant)) / (2 * a);
*(roots + 1) = (-b - sqrt(discriminant)) / (2 * a);
} else if (discriminant == 0) { // 有一个重复的实根
*roots = -b / (2 * a);
} else { // 无实根,复数根
double realPart = -b / (2 * a), imaginaryPart = sqrt(-discriminant) / (2 * a);
*roots = complex(realPart, imaginaryPart);
*(roots + 1) = complex(realPart, -imaginaryPart); // C未内置复数类型,这里假设有个自定义complex类型
}
}
```
请注意,以上代码假设了用户已经实现了`complex`类型的自定义结构体或使用了外部库来表示复数。如果你只需要求实数根,那么不需要考虑复数部分。
阅读全文
相关推荐

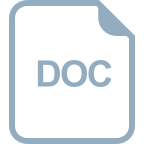













