将qimage图片转为avframe
时间: 2024-10-09 07:03:11 浏览: 102
将 QImage 类型的图像转换为 AVFrame 是一种常见的多媒体处理任务,在处理视频流时会遇到。QImage 是 Qt 库中用于表示位图图像的数据结构,而 AVFrame 是 FFmpeg 中用于表示视频帧的数据结构。以下是将 QImage 转换为 AVFrame 的步骤:
1. 首先,你需要包含必要的头文件:
```cpp
#include <opencv2/opencv.hpp>
#include <libavcodec/avcodec.h>
#include <libavformat/avformat.h>
```
2. 初始化 FFmpeg 编解码器和帧:
```cpp
AVCodec *codec = nullptr;
AVFormatContext *fmt_ctx = nullptr;
AVFrame *frame = av_frame_alloc();
```
3. 创建 QImage 到 YUV 或 RGB 数据的转换(这里假设是 YUV 因为 FFmpeg 默认是 YUV 格式):
```cpp
cv::Mat image_mat = cv::imread("path_to_your_qimage.png", cv::IMREAD_COLOR);
uchar* yuv_data = new uchar[3 * frame->height * frame->width]; // assuming 4:2:0 planar format
```
4. 使用 OpenCV 将 QImage 转换为 YUV 并复制到 `yuv_data`:
```cpp
int width = image_mat.cols;
int height = image_mat.rows;
int y_idx = 0, u_idx = height * width, v_idx = u_idx + (height / 2) * width;
// Convert to YUV and copy data
for (int row = 0; row < height; ++row) {
for (int col = 0; col < width; ++col) {
int bgra = image_mat.at<cv::Vec4b>(row, col)[2] << 16 | image_mat.at<cv::Vec4b>(row, col)[1] << 8 | image_mat.at<cv::Vec4b>(row, col)[0];
yuv_data[y_idx++] = ((bgra >> 16) & 0xFF) * 0.299f + ((bgra >> 8) & 0xFF) * 0.587f + (bgra & 0xFF) * 0.114f;
if (row % 2 == 0 && col % 2 != 0)
u_idx += (bgra >> 16) & 0xFF;
else if (row % 2 != 0 && col % 2 == 0)
v_idx += (bgra >> 16) & 0xFF;
}
}
```
5. 设置 AVFrame 的尺寸、数据和格式:
```cpp
frame->data[0] = yuv_data;
frame->linesize[0] = frame->width;
frame->format = AV_PIX_FMT_YUV420P; // Assuming 4:2:0 YUV
frame->width = width;
frame->height = height;
```
6. 清理内存并关闭资源:
```cpp
delete[] yuv_data;
if (codec)
avcodec_free_context(&codec);
if (fmt_ctx)
avformat_close_input(&fmt_ctx);
```
阅读全文
相关推荐
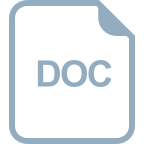
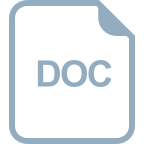
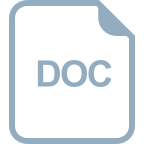















