多层lstm pytorch实现
时间: 2023-11-13 20:02:45 浏览: 45
好的,关于多层LSTM的PyTorch实现,可以按照以下步骤进行:
1. 导入必要的库和模块:
```python
import torch
import torch.nn as nn
```
2. 定义LSTM模型类:
```python
class LSTMModel(nn.Module):
def __init__(self, input_size, hidden_size, num_layers, output_size):
super(LSTMModel, self).__init__()
self.hidden_size = hidden_size
self.num_layers = num_layers
self.lstm = nn.LSTM(input_size, hidden_size, num_layers, batch_first=True)
self.fc = nn.Linear(hidden_size, output_size)
def forward(self, x):
h0 = torch.zeros(self.num_layers, x.size(0), self.hidden_size).to(x.device)
c0 = torch.zeros(self.num_layers, x.size(0), self.hidden_size).to(x.device)
out, _ = self.lstm(x, (h0, c0))
out = self.fc(out[:, -1, :])
return out
```
其中,`input_size`表示输入数据的特征数,`hidden_size`表示LSTM隐藏层的大小,`num_layers`表示LSTM的层数,`output_size`表示输出数据的大小。
3. 实例化模型:
```python
model = LSTMModel(input_size=10, hidden_size=20, num_layers=2, output_size=1)
```
其中,`input_size`为10,表示输入数据有10个特征;`hidden_size`为20,表示LSTM隐藏层有20个神经元;`num_layers`为2,表示LSTM有2层;`output_size`为1,表示输出数据的大小为1。
4. 定义损失函数和优化器:
```python
criterion = nn.MSELoss()
optimizer = torch.optim.Adam(model.parameters(), lr=0.01)
```
其中,使用均方误差(MSE)作为损失函数,使用Adam优化器进行优化。
5. 训练模型:
```python
for epoch in range(num_epochs):
for i, (inputs, labels) in enumerate(train_loader):
inputs = inputs.to(device)
labels = labels.to(device)
outputs = model(inputs)
loss = criterion(outputs, labels)
optimizer.zero_grad()
loss.backward()
optimizer.step()
if (i+1) % 10 == 0:
print('Epoch [{}/{}], Step [{}/{}], Loss: {:.4f}'
.format(epoch+1, num_epochs, i+1, total_step, loss.item()))
```
其中,`train_loader`是训练数据的数据加载器,`num_epochs`表示训练的轮数,`total_step`表示每轮训练的总步数。
6. 测试模型:
```python
with torch.no_grad():
correct = 0
total = 0
for inputs, labels in test_loader:
inputs = inputs.to(device)
labels = labels.to(device)
outputs = model(inputs)
_, predicted = torch.max(outputs.data, 1)
total += labels.size(0)
correct += (predicted == labels).sum().item()
print('Test Accuracy of the model on the {} test images: {} %'.format(total, 100 * correct / total))
```
其中,`test_loader`是测试数据的数据加载器。
相关推荐
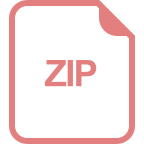
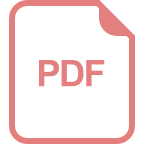
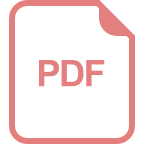














