请使用蒙特卡洛方法重新写一个c语言程序
时间: 2024-10-24 09:14:46 浏览: 7
蒙特卡洛方法是一种统计模拟方法,它通过大量随机试验来解决复杂问题。让我们用C语言重新编写一个简单示例,比如估算圆周率π。通常,我们会利用圆内点的数量与总面积的比例来逼近π。下面是基于蒙特卡罗方法估计π的一个例子:
```c
#include <stdio.h>
#include <stdlib.h>
#include <time.h>
#define RADIUS 1
#define SQUARE_SIDE 4
#define NUM_TESTS 1000000
// 用于记录落在圆内的点数量
int num_points_in_circle = 0;
// 生成随机坐标
void generate_random_point(int x, int y) {
static const double random_range = (double) SQUARE_SIDE;
x = rand() % (random_range + 1);
y = rand() % (random_range + 1);
}
// 检查随机点是否在圆内
int is_point_in_circle(int x, int y) {
int distance_squared = x * x + y * y;
return distance_squared <= RADIUS * RADIUS;
}
int main() {
srand(time(NULL));
for (int i = 0; i < NUM_TESTS; i++) {
generate_random_point(x, y);
if (is_point_in_circle(x, y)) {
num_points_in_circle++;
}
}
double estimated_pi = 4.0 * ((double) num_points_in_circle / NUM_TESTS);
printf("Estimated pi using Monte Carlo method: %.6f\n", estimated_pi);
return 0;
}
```
这个程序会随机生成大量的点,并检查它们是否位于正方形区域(边长为4单位,包含圆)内。由于圆的面积大约是正方形的一半,而落在圆内的点比例接近于π/4,通过多次试验,我们可以估算出π的值。
阅读全文
相关推荐
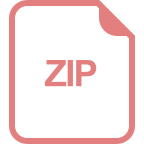
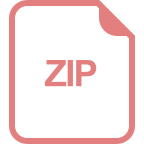

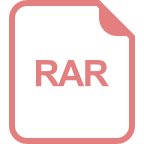
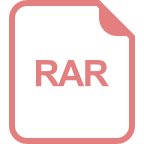
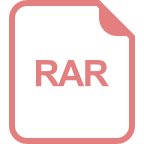
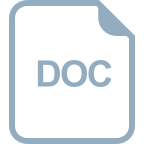








