写一个用数组使用蒙特卡洛树的亚马逊棋的C语言程序
时间: 2023-11-24 13:06:10 浏览: 31
以下是使用数组实现蒙特卡洛树搜索的亚马逊棋C语言程序:
```c
#include <stdio.h>
#include <stdlib.h>
#include <time.h>
#define BOARD_SIZE 10
#define EMPTY 0
#define WHITE 1
#define BLACK 2
int board[BOARD_SIZE][BOARD_SIZE]; // 棋盘
int player; // 当前玩家
int get_opponent(int player) {
return player == WHITE ? BLACK : WHITE;
}
int is_valid_position(int x, int y) {
return x >= 0 && x < BOARD_SIZE && y >= 0 && y < BOARD_SIZE;
}
int is_empty(int x, int y) {
return board[x][y] == EMPTY;
}
int is_same_color(int x, int y, int color) {
return board[x][y] == color;
}
int is_adjacent(int x1, int y1, int x2, int y2) {
return abs(x1 - x2) <= 1 && abs(y1 - y2) <= 1;
}
int is_valid_move(int x1, int y1, int x2, int y2) {
if (!is_valid_position(x1, y1) || !is_valid_position(x2, y2)) {
return 0;
}
if (!is_empty(x2, y2)) {
return 0;
}
if (!is_same_color(x1, y1, player)) {
return 0;
}
if (is_same_color(x2, y2, player)) {
return 0;
}
if (!is_adjacent(x1, y1, x2, y2)) {
return 0;
}
return 1;
}
void print_board() {
for (int i = 0; i < BOARD_SIZE; i++) {
for (int j = 0; j < BOARD_SIZE; j++) {
printf("%d ", board[i][j]);
}
printf("\n");
}
}
void init_board() {
for (int i = 0; i < BOARD_SIZE; i++) {
for (int j = 0; j < BOARD_SIZE; j++) {
if (i < 4 && j < 4) {
board[i][j] = BLACK;
} else if (i >= BOARD_SIZE - 4 && j >= BOARD_SIZE - 4) {
board[i][j] = WHITE;
} else {
board[i][j] = EMPTY;
}
}
}
}
int get_random_move(int *x1, int *y1, int *x2, int *y2) {
int valid_moves[BOARD_SIZE * BOARD_SIZE][4];
int num_moves = 0;
for (int i = 0; i < BOARD_SIZE; i++) {
for (int j = 0; j < BOARD_SIZE; j++) {
if (is_same_color(i, j, player)) {
for (int k = i - 1; k <= i + 1; k++) {
for (int l = j - 1; l <= j + 1; l++) {
if (is_valid_move(i, j, k, l)) {
valid_moves[num_moves][0] = i;
valid_moves[num_moves][1] = j;
valid_moves[num_moves][2] = k;
valid_moves[num_moves][3] = l;
num_moves++;
}
}
}
}
}
}
if (num_moves == 0) {
return 0;
}
int rand_index = rand() % num_moves;
*x1 = valid_moves[rand_index][0];
*y1 = valid_moves[rand_index][1];
*x2 = valid_moves[rand_index][2];
*y2 = valid_moves[rand_index][3];
return 1;
}
int simulate() {
int winner = EMPTY;
while (1) {
int x1, y1, x2, y2;
if (!get_random_move(&x1, &y1, &x2, &y2)) {
break;
}
board[x2][y2] = player;
board[x1][y1] = EMPTY;
player = get_opponent(player);
}
int num_black = 0, num_white = 0;
for (int i = 0; i < BOARD_SIZE; i++) {
for (int j = 0; j < BOARD_SIZE; j++) {
if (board[i][j] == BLACK) {
num_black++;
} else if (board[i][j] == WHITE) {
num_white++;
}
}
}
if (num_black > num_white) {
winner = BLACK;
} else if (num_white > num_black) {
winner = WHITE;
}
return winner;
}
double monte_carlo_tree_search(int num_iterations) {
int num_wins = 0;
for (int i = 0; i < num_iterations; i++) {
int board_copy[BOARD_SIZE][BOARD_SIZE];
for (int j = 0; j < BOARD_SIZE; j++) {
for (int k = 0; k < BOARD_SIZE; k++) {
board_copy[j][k] = board[j][k];
}
}
int player_copy = player;
int winner = simulate();
if (winner == player_copy) {
num_wins++;
}
player = player_copy;
for (int j = 0; j < BOARD_SIZE; j++) {
for (int k = 0; k < BOARD_SIZE; k++) {
board[j][k] = board_copy[j][k];
}
}
}
return (double)num_wins / num_iterations;
}
int main() {
srand(time(NULL));
init_board();
player = BLACK;
print_board();
while (1) {
int x1, y1, x2, y2;
if (!get_random_move(&x1, &y1, &x2, &y2)) {
break;
}
printf("Player %d moves from (%d, %d) to (%d, %d)\n", player, x1, y1, x2, y2);
board[x2][y2] = player;
board[x1][y1] = EMPTY;
player = get_opponent(player);
print_board();
double win_rate = monte_carlo_tree_search(1000);
printf("Win rate: %.2f\n", win_rate);
}
return 0;
}
```
该程序使用蒙特卡洛树搜索来预测每个玩家的胜率,并选择具有最高胜率的动作。在每个回合结束时,程序会打印胜率和当前棋盘状态。
相关推荐
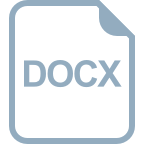
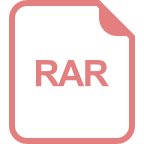














