写一个亚马逊棋蒙特卡洛树
时间: 2024-05-04 11:19:00 浏览: 175
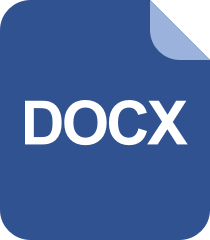
关于亚马逊棋蒙特卡洛博弈算法的并行优化的综述.docx
亚马逊棋是一种棋类游戏,玩家需要在棋盘上放置障碍物并控制棋子移动,以消灭对手的棋子。Monte Carlo Tree Search(MCTS)是一种常用的人工智能算法,可用于解决许多类似棋类游戏的问题。在这里,我们将使用MCTS算法来解决亚马逊棋。
首先,我们需要定义棋盘和棋子的状态。我们可以使用一个二维数组来表示棋盘,其中0表示空的位置,1表示玩家1的棋子,2表示玩家2的棋子,3表示障碍物。我们还需要跟踪当前哪位玩家正在操作,以及哪些位置可以放置障碍物。
接下来,我们需要定义MCTS算法的几个组成部分。首先是模拟阶段,其中我们会选择一个随机动作并模拟其结果。
```
def simulate(board, player):
actions = get_possible_actions(board, player)
if len(actions) == 0:
return -1
action = random.choice(actions)
result = execute_action(board, player, action)
if result == player:
return 1
elif result == -1:
return 0
else:
return -simulate(board, 3 - player)
```
在这里,我们首先获取当前玩家可以执行的所有操作,然后随机选择一个操作并模拟其结果。如果结果是当前玩家赢了,我们返回1;如果结果是平局,我们返回0;否则,我们继续模拟对手的动作并返回其相反数。
接下来是选择阶段,在这里我们会选择一个具有最大置信上限的子节点进行扩展。
```
def select(node):
while not node.is_leaf():
node = max(node.children, key=uct_score)
return node
```
在这里,我们一直向下遍历树,直到找到一个叶节点。在遍历过程中,我们会计算每个子节点的置信上限得分,然后选择得分最高的子节点进行扩展。
接下来是扩展阶段,在这里我们会添加一个新节点并执行一个随机动作。
```
def expand(node):
actions = get_possible_actions(node.board, node.player)
action = random.choice(actions)
new_board = execute_action(node.board, node.player, action)
new_player = 3 - node.player
new_node = Node(new_board, new_player, node)
node.children.append(new_node)
return new_node
```
在这里,我们首先获取当前玩家可以执行的所有操作,然后随机选择一个操作并模拟其结果。我们将新棋盘状态添加到树中作为一个新的节点,然后将该节点添加到当前节点的子节点列表中。
最后是回溯阶段,在这里我们会将模拟结果传播到根节点。
```
def backpropagate(node, result):
while node is not None:
node.visits += 1
node.wins += result
node = node.parent
```
在这里,我们会将模拟结果传播到当前节点的所有祖先节点。我们还会增加每个节点的访问计数和胜利计数。
现在我们可以将所有这些组成部分合并为一个完整的MCTS算法。
```
class Node:
def __init__(self, board, player, parent=None):
self.board = board
self.player = player
self.parent = parent
self.children = []
self.wins = 0
self.visits = 0
def is_leaf(self):
return len(self.children) == 0
def uct_score(node):
if node.visits == 0:
return float('inf')
return node.wins / node.visits + math.sqrt(2 * math.log(node.parent.visits) / node.visits)
def get_possible_actions(board, player):
actions = []
for i in range(10):
for j in range(10):
if board[i][j] == player:
for di, dj in [(1, 0), (-1, 0), (0, 1), (0, -1)]:
ni, nj = i + di, j + dj
while 0 <= ni < 10 and 0 <= nj < 10:
if board[ni][nj] == 0:
actions.append((i, j, ni, nj))
ni, nj = ni + di, nj + dj
else:
break
if len(actions) == 0:
actions.append((-1, -1, -1, -1))
return actions
def execute_action(board, player, action):
if action == (-1, -1, -1, -1):
return -1
i, j, ni, nj = action
if board[ni][nj] == 3:
return 3 - player
board[i][j] = 0
board[ni][nj] = player
for di, dj in [(1, 0), (-1, 0), (0, 1), (0, -1)]:
ni, nj = i + di, j + dj
while 0 <= ni < 10 and 0 <= nj < 10 and board[ni][nj] == 0:
board[ni][nj] = 3
ni, nj = ni + di, nj + dj
return 0
def simulate(board, player):
actions = get_possible_actions(board, player)
if len(actions) == 0:
return -1
action = random.choice(actions)
result = execute_action(board, player, action)
if result == player:
return 1
elif result == -1:
return 0
else:
return -simulate(board, 3 - player)
def select(node):
while not node.is_leaf():
node = max(node.children, key=uct_score)
return node
def expand(node):
actions = get_possible_actions(node.board, node.player)
action = random.choice(actions)
new_board = execute_action(node.board, node.player, action)
new_player = 3 - node.player
new_node = Node(new_board, new_player, node)
node.children.append(new_node)
return new_node
def backpropagate(node, result):
while node is not None:
node.visits += 1
node.wins += result
node = node.parent
def mcts(board, player, iterations):
root = Node(board, player)
for i in range(iterations):
node = select(root)
if node.visits == 0:
result = simulate(node.board, node.player)
else:
node = expand(node)
result = simulate(node.board, node.player)
backpropagate(node, result)
return max(root.children, key=lambda node: node.visits).board
```
在这里,我们定义了一个MCTS函数,其中我们指定当前棋盘状态、当前玩家以及要运行的迭代次数。我们首先创建一个根节点,然后执行指定数量的迭代。在每次迭代中,我们选择一个节点进行扩展或模拟,并将结果传播回根节点。最后,我们返回访问计数最高的子节点的棋盘状态作为我们的决策。
现在我们可以使用这个MCTS算法来解决亚马逊棋问题。
阅读全文
相关推荐
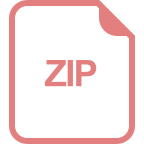















