y用c语言计算多少个月还清贷款
时间: 2024-10-21 17:16:10 浏览: 30
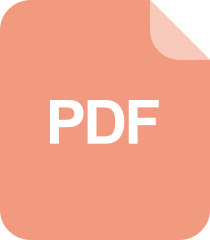
C语言小程序 如何判断两个日期之差
在C语言中,要计算清偿贷款所需的月数,我们需要了解一些基本的数学公式和变量。假设我们有以下几个变量:
1. `total_amount`:贷款总额。
2. `monthly_payment`:每月偿还额。
3. `interest_rate`:年利率(通常作为小数表示)。
4. `loan_term_in_years`:贷款年限(以年为单位)。
首先,我们需要将年利率转换为月利率。然后,我们可以利用等额本息还款法(也称为固定利率还款法)计算出需要的月数。具体的计算步骤如下:
1. 计算月利率:`monthly_interest_rate = interest_rate / 100 / 12`
2. 如果每月支付的利息大于剩余本金乘以月利率,则采用"部分本金+部分利息"的方式还款,直到全部还清;否则,只付利息。
3. 设置初始余额为总金额:`current_balance = total_amount`
4. 设定一个计数器 `months_to_payback` 初始化为0
5. 循环计算月数,直到当前余额为0为止:
a. 按月计算利息:`interest = current_balance * monthly_interest_rate`
b. 还款本金:`principal_paid = monthly_payment - interest`
c. 更新当前余额:`current_balance -= principal_paid`
d. 提高计数器:`months_to_payback++`
最后,`months_to_payback` 就是清偿贷款所需的月数。
```c
#include <stdio.h>
double calculate_months(double total_amount, double monthly_payment, double interest_rate, int loan_term_in_years) {
double monthly_interest_rate = interest_rate / 100 / 12;
double current_balance = total_amount;
int months_to_payback = 0;
while (current_balance > 0) {
double interest = current_balance * monthly_interest_rate;
double principal_paid = monthly_payment - interest;
current_balance -= principal_paid;
months_to_payback++;
}
return months_to_payback;
}
int main() {
double total_amount, monthly_payment, interest_rate, loan_term_in_years;
// 输入贷款相关数值...
printf("贷款月数:%.2f\n", calculate_months(total_amount, monthly_payment, interest_rate, loan_term_in_years));
return 0;
}
```
阅读全文
相关推荐
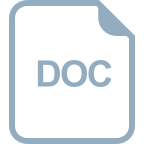
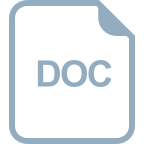
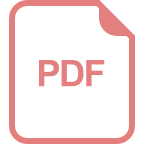













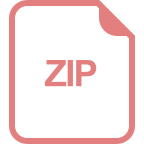
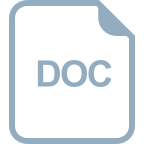