输出自然数1到n所有不重复的排列
时间: 2023-08-09 07:00:41 浏览: 100
要输出自然数1到n的所有不重复排列,可以使用递归方法进行求解。
在每一层递归中,首先确定一个数作为当前排列的第一个数,然后在剩余的数中递归求解剩下的排列。具体步骤如下:
1. 定义一个数组perms,用于存储所有的不重复排列。
2. 编写一个递归函数generatePermutations,接受三个参数:当前要生成的排列permutation,当前可选的数字candidates,以及perms数组。
3. 终止条件:当candidates为空时,表示已经用完了所有的数字,此时将当前的排列permutation加入到perms数组中,并返回。
4. 在递归函数内部,遍历所有的可选数字candidates。
5. 将当前数字从candidates中移除,并将其加入到当前排列permutation后面。
6. 递归调用generatePermutations函数,将当前排列permutation和更新后的candidates作为参数传入。
7. 在递归函数返回后,将当前数字从permutation中移除,恢复candidates数组原来的状态。
8. 返回perms数组,即为所有不重复排列的结果。
以下是示例代码实现:
```python
def generatePermutations(permutation, candidates, perms):
if len(candidates) == 0:
perms.append(permutation)
return
for i in range(len(candidates)):
num = candidates[i]
generatePermutations(permutation + [num], candidates[:i] + candidates[i+1:], perms)
def getPermutations(n):
candidates = list(range(1, n+1))
perms = []
generatePermutations([], candidates, perms)
return perms
n = 3
permutations = getPermutations(n)
for perm in permutations:
print(perm)
```
以上代码可以输出自然数1到n的所有不重复排列。
阅读全文
相关推荐













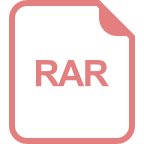

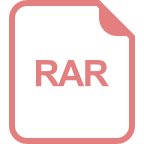