使用 C 语言实现:使用结构体和指针存储线性表结构求链表节点总数、添加新节点、输出所有节点、任意位置插入新节点、删除节 点、查找节点
时间: 2024-09-27 08:04:36 浏览: 14
在C语言中,可以使用结构体和指针来实现链表的基本操作。下面是一些关键步骤:
1. **定义结构体(Node)**:
```c
typedef struct Node {
int data; // 节点数据
struct Node* next; // 指向下一个节点的指针
} Node;
```
2. **计算节点总数(链表长度)**:
```c
int count_nodes(Node* head) {
int count = 0;
if (head != NULL) {
Node* current = head;
while (current != NULL) {
count++;
current = current->next;
}
}
return count;
}
```
3. **添加新节点**:
```c
void add_node(Node** head, int data, int position) {
Node* newNode = (Node*)malloc(sizeof(Node));
newNode->data = data;
newNode->next = *head;
if (position == 0) {
*head = newNode;
} else {
Node* prev = *head;
for (int i = 1; i < position - 1 && prev != NULL; i++) {
prev = prev->next;
}
if (prev != NULL) {
newNode->next = prev->next;
prev->next = newNode;
}
}
}
```
4. **输出所有节点**:
```c
void print_list(Node* head) {
if (head == NULL) {
printf("List is empty.\n");
} else {
Node* current = head;
while (current != NULL) {
printf("%d -> ", current->data);
current = current->next;
}
printf("NULL\n");
}
}
```
5. **任意位置插入新节点**:
已包含在上述`add_node`函数中。
6. **删除节点**:
```c
void delete_node(Node** head, int data) {
if (*head == NULL) {
return;
}
Node* current = *head;
if (current->data == data) {
*head = current->next;
free(current);
return;
}
Node* prev = current;
while (current->next != NULL && current->data != data) {
prev = current;
current = current->next;
}
if (current->data == data) {
prev->next = current->next;
free(current);
}
}
```
7. **查找节点**:
```c
Node* search_node(Node* head, int data) {
Node* current = head;
while (current != NULL) {
if (current->data == data) {
return current;
}
current = current->next;
}
return NULL;
}
```
相关推荐
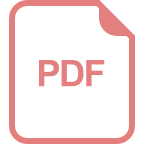
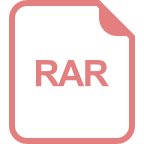
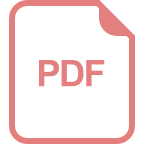














