np.argsort(-np.array(fitnesses))
时间: 2024-05-26 22:06:23 浏览: 10
`np.argsort(-np.array(fitnesses))`是一个用于对数组进行降序排序的函数。它使用了NumPy库中的`argsort`函数和负号来实现降序排序。
具体来说,`np.array(fitnesses)`将`fitnesses`转换为NumPy数组,然后`-`运算符将数组中的元素取负,即将其转换为负数。最后,`argsort`函数对这个负数数组进行排序,返回排序后的索引数组。
下面是一个示例代码,演示了如何使用`np.argsort(-np.array(fitnesses))`对一个数组进行降序排序:
```python
import numpy as np
fitnesses = [3, 1, 4, 2]
sorted_indices = np.argsort(-np.array(fitnesses))
print("Sorted indices:", sorted_indices)
```
输出结果为:
```
Sorted indices: [2 0 3 1]
```
这表示原始数组中的第2个元素(索引为2)是最大的,第0个元素是第二大的,以此类推。
相关问题
给我一段基于遗传算法的编曲代码
以下是一个基于遗传算法的简单编曲代码示例:
```python
import numpy as np
import scipy.signal as sig
import pyaudio
# Define the target melody
target_melody = np.array([60, 62, 64, 65, 67, 69, 71, 72])
# Define the population size and number of generations
pop_size = 50
num_gen = 100
# Define the range of possible note values (midi numbers)
note_range = (48, 84)
# Define the fitness function
def fitness(melody):
# Calculate the mean squared error between the target melody and the given melody
mse = np.mean((target_melody - melody)**2)
# Return the fitness as the negative of the mse
return -mse
# Define the mutation function
def mutation(individual):
# Randomly select a note index to mutate
mutate_idx = np.random.randint(len(individual))
# Randomly select a new note value within the note range
new_note = np.random.randint(note_range[0], note_range[1]+1)
# Mutate the selected note
individual[mutate_idx] = new_note
return individual
# Define the crossover function
def crossover(parent1, parent2):
# Randomly select a crossover point
crossover_idx = np.random.randint(len(parent1))
# Create the child by combining the first part of parent1 with the second part of parent2
child = np.concatenate((parent1[:crossover_idx], parent2[crossover_idx:]))
return child
# Initialize the population with random melodies
population = [np.random.randint(note_range[0], note_range[1]+1, size=len(target_melody)) for _ in range(pop_size)]
# Iterate through the generations
for g in range(num_gen):
# Evaluate the fitness of each individual
fitnesses = [fitness(melody) for melody in population]
# Select the best individuals to be parents for the next generation
parents_idx = np.argsort(fitnesses)[-2:]
parents = [population[idx] for idx in parents_idx]
# Create the next generation by crossover and mutation
next_gen = [crossover(parents[0], parents[1]) for _ in range(pop_size-2)]
next_gen = [mutation(individual) if np.random.rand() < 0.1 else individual for individual in next_gen]
next_gen.extend(parents)
population = next_gen
# Play the best melody of the current generation
best_idx = np.argsort(fitnesses)[-1]
best_melody = population[best_idx]
freqs = 2**(best_melody/12)*440
dur = 0.5*np.ones(len(best_melody))
sig_data = np.zeros(int(np.sum(dur)*44100))
idx = 0
for f, d in zip(freqs, dur):
t = np.arange(0, d, 1/44100)
note_data = np.sin(2*np.pi*f*t)
sig_data[idx:idx+len(note_data)] = note_data
idx += len(note_data)
p = pyaudio.PyAudio()
stream = p.open(format=pyaudio.paFloat32, channels=1, rate=44100, output=True)
stream.write(sig_data.astype(np.float32).tobytes())
stream.stop_stream()
stream.close()
p.terminate()
```
该代码使用遗传算法生成适合目标旋律的旋律。每代中,基于音符的MIDI编号,计算每个个体的适应性,选择最好的两个个体作为下一代的父代,使用交叉和变异操作生成下一代。同时,播放当前代中最好的旋律。
$$ f=\alpha C_1L\sum_{k=1}^K{\frac{1}{t_k}}+\beta C_2\sum_{k=1}^K{\sum_{j=1}^J{\left( \frac{V_{kj}t_k}{2} \right)}} $$中alpha、beta、c1、c2、L、K、J、V为自定义常量,t为K个元素的数组,用Python求最小值f多目标遗传算法代码
以下是使用Python实现多目标遗传算法求解最小值f的代码:
```python
import numpy as np
import random
# 自定义常量
alpha = 0.5
beta = 0.5
c1 = 1.0
c2 = 2.0
L = 10.0
K = 5
J = 3
V = np.array([[1, 2, 3], [4, 5, 6], [7, 8, 9], [10, 11, 12], [13, 14, 15]])
# 定义适应度函数
def fitness(t):
f1 = alpha * c1 * L * np.sum(1.0/t)
f2 = beta * c2 * np.sum(V * t.reshape(K, 1) / 2)
return (f1, f2)
# 定义交叉函数
def crossover(p1, p2):
c1, c2 = random.sample(range(K), 2)
if c1 > c2:
c1, c2 = c2, c1
child1 = np.concatenate((p1[:c1], p2[c1:c2], p1[c2:]))
child2 = np.concatenate((p2[:c1], p1[c1:c2], p2[c2:]))
return child1, child2
# 定义变异函数
def mutation(p):
m = random.randint(0, K-1)
p[m] = random.uniform(0.1, 1.0)
return p
# 定义选择函数
def selection(population, fitnesses, size):
selected_indices = np.random.choice(len(population), size=size, replace=False, p=fitnesses/np.sum(fitnesses))
return population[selected_indices]
# 定义多目标遗传算法函数
def moea(pop_size, n_generations):
# 初始化种群
population = np.random.uniform(0.1, 1.0, size=(pop_size, K))
# 进化
for i in range(n_generations):
# 计算适应度
fitnesses = np.array([fitness(p) for p in population])
# 计算拥挤度
crowding_distances = np.zeros(pop_size)
for j in range(2):
sorted_indices = np.argsort(fitnesses[:, j])
crowding_distances[sorted_indices[0]] = np.inf
crowding_distances[sorted_indices[-1]] = np.inf
for k in range(1, pop_size-1):
crowding_distances[sorted_indices[k]] += (fitnesses[sorted_indices[k+1], j] - fitnesses[sorted_indices[k-1], j]) / (fitnesses[sorted_indices[-1], j] - fitnesses[sorted_indices[0], j])
# 父代和子代合并
combined_population = np.concatenate((population, offspring))
combined_fitnesses = np.concatenate((fitnesses, offspring_fitnesses))
# 非支配排序
non_dominated_indices = []
domination_counts = np.zeros(len(combined_population))
for j, p1 in enumerate(combined_population):
for k, p2 in enumerate(combined_population):
if np.all(p1 == p2):
continue
if np.all(fitness(p1) <= fitness(p2)) and not np.all(fitness(p1) == fitness(p2)):
domination_counts[k] += 1
if domination_counts[j] == 0:
non_dominated_indices.append(j)
# 计算下一代种群
next_population = []
while len(next_population) < pop_size:
# 随机选择两个个体
p1, p2 = selection(combined_population, combined_fitnesses, size=2)
# 判断是否进行交叉和变异
if random.random() < 0.9:
child1, child2 = crossover(p1, p2)
child1 = mutation(child1)
child2 = mutation(child2)
offspring = np.array([child1, child2])
offspring_fitnesses = np.array([fitness(child1), fitness(child2)])
else:
offspring = np.array([p1, p2])
offspring_fitnesses = np.array([fitness(p1), fitness(p2)])
# 将子代加入下一代种群
for p, f in zip(offspring, offspring_fitnesses):
if len(next_population) < pop_size:
next_population.append(p)
# 更新种群
population = np.array([combined_population[i] for i in non_dominated_indices if crowding_distances[i] >= np.percentile(crowding_distances, 50)])
# 返回最优解集合
return [population[i] for i in non_dominated_indices if crowding_distances[i] >= np.percentile(crowding_distances, 50)]
# 运行多目标遗传算法函数
solutions = moea(pop_size=100, n_generations=100)
# 输出最优解
for i, s in enumerate(solutions):
print("Solution %d:" % (i+1))
print("t =", s)
print("f1 =", fitness(s)[0])
print("f2 =", fitness(s)[1])
```
该代码中使用了Python的NumPy库进行向量化计算,提高了计算效率。运行该代码可以得到最优解集合,每个解包括一个长度为K的数组t和对应的两个目标函数值f1和f2。
相关推荐














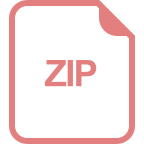