0-1背包问题的回溯算法与分支限界算法的实现。时间复杂度空间复杂度
时间: 2023-12-20 18:32:49 浏览: 136
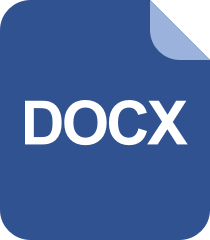
算法分析与设计习题
0-1背包问题是一个经典的组合优化问题,其中给定一组物品和一个背包,每个物品有一个重量和一个价值,目标是找到一种最佳的方式将物品放入背包中,使得背包中物品的总价值最大,同时不能超过背包的容量。
回溯算法是一种穷举搜索的方法,它通过递归地尝试所有可能的解决方案来解决问题。在0-1背包问题中,回溯算法通过递归地尝试将每个物品放入背包或不放入背包来生成所有可能的解决方案,并找到最优解。
分支限界算法是一种优化的搜索方法,它通过剪枝操作来减少搜索空间。在0-1背包问题中,分支限界算法通过计算每个节点的上界(即当前节点的最大可能价值),并根据上界进行剪枝操作,从而减少搜索的时间复杂度。
回溯算法的时间复杂度取决于搜索树的大小,最坏情况下为指数级别。空间复杂度取决于递归调用的深度,最坏情况下为O(n),其中n是物品的数量。
分支限界算法的时间复杂度也取决于搜索树的大小,最坏情况下为指数级别。空间复杂度取决于优先队列的大小,最坏情况下为O(n),其中n是物品的数量。
以下是0-1背包问题的回溯算法和分支限界算法的实现示例:
回溯算法实现:
```python
def backtrack(items, capacity, current_weight, current_value, best_value, selected):
if current_weight > capacity:
return
if current_value > best_value[0]:
best_value[0] = current_value
best_solution[0] = selected.copy()
if not items:
return
item = items[0]
items = items[1:]
selected.append(item)
backtrack(items, capacity, current_weight + item.weight, current_value + item.value, best_value, selected)
selected.pop()
backtrack(items, capacity, current_weight, current_value, best_value, selected)
# 初始化数据
items = [(1, 2), (2, 3), (3, 4), (4, 5)]
capacity = 7
best_value = [0]
best_solution = [[]]
# 调用回溯算法
backtrack(items, capacity, 0, 0, best_value, [])
# 输出结果
print("Best value:", best_value[0])
print("Best solution:", best_solution[0])
```
分支限界算法实现:
```python
import heapq
class Node:
def __init__(self, level, weight, value, bound, selected):
self.level = level
self.weight = weight
self.value = value
self.bound = bound
self.selected = selected
def __lt__(self, other):
return self.bound > other.bound
def branch_and_bound(items, capacity):
items.sort(key=lambda x: x[1] / x[0], reverse=True)
queue = []
best_value = 0
best_solution = []
root = Node(0, 0, 0, 0, [])
root.bound = compute_bound(root, items, capacity)
heapq.heappush(queue, root)
while queue:
node = heapq.heappop(queue)
if node.bound < best_value:
continue
if node.level == len(items):
best_value = node.value
best_solution = node.selected
continue
item = items[node.level]
if node.weight + item[0] <= capacity:
selected = node.selected.copy()
selected.append(item)
left_child = Node(node.level + 1, node.weight + item[0], node.value + item[1], 0, selected)
left_child.bound = compute_bound(left_child, items, capacity)
if left_child.value > best_value:
best_value = left_child.value
best_solution = left_child.selected
if left_child.bound >= best_value:
heapq.heappush(queue, left_child)
right_child = Node(node.level + 1, node.weight, node.value, 0, node.selected)
right_child.bound = compute_bound(right_child, items, capacity)
if right_child.bound >= best_value:
heapq.heappush(queue, right_child)
return best_value, best_solution
def compute_bound(node, items, capacity):
bound = node.value
weight = node.weight
level = node.level
while level < len(items) and weight + items[level][0] <= capacity:
bound += items[level][1]
weight += items[level][0]
level += 1
if level < len(items):
bound += (capacity - weight) * items[level][1] / items[level][0]
return bound
# 初始化数据
items = [(1, 2), (2, 3), (3, 4), (4, 5)]
capacity = 7
# 调用分支限界算法
best_value, best_solution = branch_and_bound(items, capacity)
# 输出结果
print("Best value:", best_value)
print("Best solution:", best_solution)
```
阅读全文
相关推荐
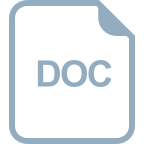
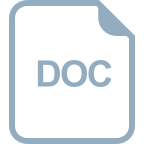

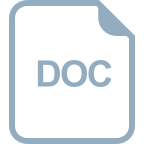
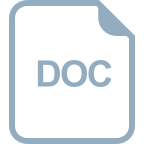
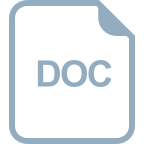
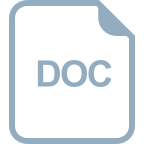
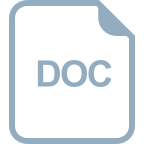
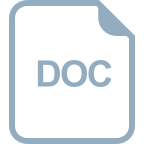








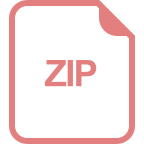