def forward(self, image): image_2 = image.permute(0, 3, 1, 2).clone() avg_pool = nn.AvgPool2d(kernel_size=2, stride=(2, 2), count_include_pad=False) cb = avg_pool(image_2[:, 1, :, :].unsqueeze(1)) cr = avg_pool(image_2[:, 2, :, :].unsqueeze(1)) cb = cb.permute(0, 2, 3, 1) cr = cr.permute(0, 2, 3, 1) return image[:, :, :, 0], cb.squeeze(3), cr.squeeze(3)解释
时间: 2023-09-30 12:07:34 浏览: 49
这是一个 PyTorch 的前向传播函数,用于从输入的图像中提取出 YCbCr 三个通道的信息。具体来说,它的输入是一个四维张量 image,表示一批图像,其形状为 (batch_size, height, width, 3),其中 3 表示 RGB 三个通道。该函数的输出包括三个张量,分别是 Y、Cb、Cr 三个通道的信息,形状分别为 (batch_size, height, width, 1)、(batch_size, height/2, width/2, 1)、(batch_size, height/2, width/2, 1)。
该函数的实现过程如下:
首先,将输入张量 image 维度的顺序从 (batch_size, height, width, 3) 转换为 (batch_size, 3, height, width)。这样做是为了方便对 RGB 三个通道进行处理。
然后,利用 nn.AvgPool2d 模块对 Cb、Cr 两个通道做 2 倍下采样。这里采用平均池化的方式进行下采样,池化核大小为 2,步长为 (2, 2)。
接着,将下采样后的 Cb、Cr 两个通道的维度顺序从 (batch_size, 1, height/2, width/2) 转换为 (batch_size, height/2, width/2, 1)。这里采用 permute 函数实现维度转换。
最后,返回 Y、Cb、Cr 三个通道的信息,其中 Y 通道直接从输入张量中取出,而 Cb、Cr 两个通道则是经过下采样后得到的。
相关问题
class BearingFaultDetectionModel(nn.Module): def __init__(self): super(BearingFaultDetectionModel, self).__init__() self.attention = nn.MultiheadAttention(embed_dim=10, num_heads=1) # 注意力层 self.dense = nn.Linear(10, 1) # 输出层,预测轴承是否存在故障 def forward(self, inputs): attention_outputs, _ = self.attention(inputs.permute(1, 0, 2), inputs.permute(1, 0, 2), inputs.permute(1, 0, 2)) # 计算注意力权重 attention_outputs = attention_outputs.permute(1, 0, 2) weighted_inputs = attention_outputs * inputs # 使用注意力权重进行加权 output = self.dense(weighted_inputs) # 输出层 return output
这是一个用于轴承故障检测的模型。它包含一个注意力层和一个输出层。
在`__init__`方法中,我们首先调用`super()`来继承父类`nn.Module`的属性和方法。然后,我们定义了一个`nn.MultiheadAttention`层作为注意力层,其中`embed_dim=10`表示输入特征的维度为10,`num_heads=1`表示只使用一个注意力头。接下来,我们定义了一个线性层`nn.Linear(10, 1)`作为输出层,用于预测轴承是否存在故障。
在`forward`方法中,我们首先通过调用注意力层`self.attention`计算输入特征的注意力权重。然后,对注意力权重和输入特征进行维度转换,并将它们相乘得到加权的输入特征`weighted_inputs`。最后,将加权的输入特征传递给输出层`self.dense`进行预测,得到输出结果。
这个模型的目标是通过引入注意力机制,对轴承故障进行检测并进行预测。注意力机制可以帮助模型更好地关注轴承特征中的重要信息,并根据注意力权重进行加权处理,进而提高检测和预测的准确性。
class STHSL(nn.Module): def __init__(self): super(STHSL, self).__init__() self.dimConv_in = nn.Conv3d(1, args.latdim, kernel_size=1, padding=0, bias=True) self.dimConv_local = nn.Conv2d(args.latdim, 1, kernel_size=1, padding=0, bias=True) self.dimConv_global = nn.Conv2d(args.latdim, 1, kernel_size=1, padding=0, bias=True) self.spa_cnn_local1 = spa_cnn_local(args.latdim, args.latdim) self.spa_cnn_local2 = spa_cnn_local(args.latdim, args.latdim) self.tem_cnn_local1 = tem_cnn_local(args.latdim, args.latdim) self.tem_cnn_local2 = tem_cnn_local(args.latdim, args.latdim) self.Hypergraph_Infomax = Hypergraph_Infomax() self.tem_cnn_global1 = tem_cnn_global(args.latdim, args.latdim, 9) self.tem_cnn_global2 = tem_cnn_global(args.latdim, args.latdim, 9) self.tem_cnn_global3 = tem_cnn_global(args.latdim, args.latdim, 9) self.tem_cnn_global4 = tem_cnn_global(args.latdim, args.latdim, 6) self.local_tra = Transform_3d() self.global_tra = Transform_3d() def forward(self, embeds_true, neg): embeds_in_global = self.dimConv_in(embeds_true.unsqueeze(1)) DGI_neg = self.dimConv_in(neg.unsqueeze(1)) embeds_in_local = embeds_in_global.permute(0, 3, 1, 2, 4).contiguous().view(-1, args.latdim, args.row, args.col, 4) spa_local1 = self.spa_cnn_local1(embeds_in_local) spa_local2 = self.spa_cnn_local2(spa_local1) spa_local2 = spa_local2.view(-1, args.temporalRange, args.latdim, args.areaNum, args.cateNum).permute(0, 2, 3, 1, 4) tem_local1 = self.tem_cnn_local1(spa_local2) tem_local2 = self.tem_cnn_local2(tem_local1) eb_local = tem_local2.mean(3) eb_tra_local = self.local_tra(tem_local2) out_local = self.dimConv_local(eb_local).squeeze(1) hy_embeds, Infomax_pred = self.Hypergraph_Infomax(embeds_in_global, DGI_neg) tem_global1 = self.tem_cnn_global1(hy_embeds) tem_global2 = self.tem_cnn_global2(tem_global1) tem_global3 = self.tem_cnn_global3(tem_global2) tem_global4 = self.tem_cnn_global4(tem_global3) eb_global = tem_global4.squeeze(3) eb_tra_global = self.global_tra(tem_global4) out_global = self.dimConv_global(eb_global).squeeze(1) return out_local, eb_tra_local, eb_tra_global, Infomax_pred, out_global
这是一个使用 PyTorch 实现的神经网络模型的定义类。在这个模型中,输入数据包含一个真实的嵌入向量和一个负样本向量。模型将这些数据作为输入,并执行一系列操作来生成输出。具体来说,模型包含了一些卷积和全连接层,以及一些变换和聚合操作。这些操作包括将嵌入向量转换为局部和全局表示,通过超图信息最大化来学习嵌入向量的表示,以及对局部和全局表示进行转换和聚合。最终,模型的输出包括局部和全局表示,超图信息最大化的预测结果,以及对局部和全局表示进行卷积后得到的输出。
相关推荐
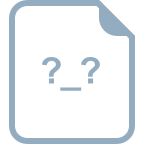
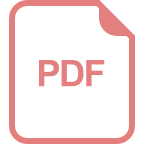













