c语言代码后缀表达式计算
时间: 2024-05-26 10:08:56 浏览: 173
C语言可以使用栈来实现后缀表达式计算。后缀表达式也叫逆波兰表达式,是一种将运算符放在后面的表达式。例如,中缀表达式3+4转换为后缀表达式为3 4 +。具体步骤如下:
1. 从左到右遍历表达式的每个元素。
2. 如果当前元素是数字,将其压入栈中。
3. 如果当前元素是操作符,从栈中弹出两个元素进行运算,然后将结果压入栈中。
4. 当遍历完整个表达式后,栈中只剩下一个元素,即为计算结果。
下面是一个C语言实现后缀表达式计算的代码示例:
```
#include <stdio.h>
#include <stdlib.h>
#include <ctype.h>
#define MAX_STACK_SIZE 100
typedef struct {
int data[MAX_STACK_SIZE];
int top;
} Stack;
void push(Stack *s, int item) {
if (s->top == MAX_STACK_SIZE - 1) {
printf("Stack overflow!\n");
exit(EXIT_FAILURE);
} else {
s->data[++(s->top)] = item;
}
}
int pop(Stack *s) {
if (s->top == -1) {
printf("Stack underflow!\n");
exit(EXIT_FAILURE);
} else {
return s->data[(s->top)--];
}
}
int is_operator(char c) {
return c == '+' || c == '-' || c == '*' || c == '/';
}
int evaluate_postfix(char *expression) {
Stack s;
s.top = -1;
while (*expression != '\0') {
if (isdigit(*expression)) {
push(&s, *expression - '0');
} else if (is_operator(*expression)) {
int operand2 = pop(&s);
int operand1 = pop(&s);
switch (*expression) {
case '+':
push(&s, operand1 + operand2);
break;
case '-':
push(&s, operand1 - operand2);
break;
case '*':
push(&s, operand1 * operand2);
break;
case '/':
push(&s, operand1 / operand2);
break;
}
}
expression++;
}
return pop(&s);
}
int main() {
char postfix_expression[] = "34+5*";
int result = evaluate_postfix(postfix_expression);
printf("Result: %d\n", result);
return 0;
}
```
阅读全文
相关推荐
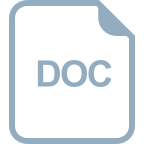

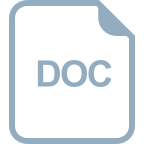


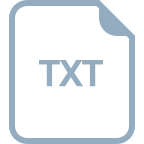
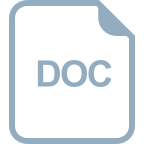
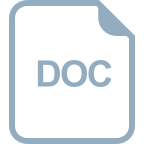
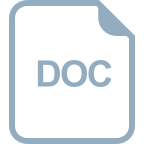



