帮我写一个程序,根据输入长度为n的n个关键字值序列,对其采用希尔排序(增量为5,3,1),冒泡排序进行从小到大排序,最后输出每趟希尔排序和冒泡排序的结果。1、希尔算法1)生成增量数组,调用单次排序函数;2)按照每次要求的增量对查找表L进行排序(直接插入排序)p272;3)打印每次希尔排序结构2、冒泡排序1)计算比较的轮次及每次需要比较的次数;2)比较相邻数组元素的大小,顺序不对即交换;3)打印每轮冒泡排序之后的序列3、主函数调用4、头文件
时间: 2024-03-12 12:44:22 浏览: 74
好的,以下是程序代码:
头文件 shell_bubble_sort.h:
```cpp
#ifndef SHELL_BUBBLE_SORT_H
#define SHELL_BUBBLE_SORT_H
void shellSort(int arr[], int n);
void bubbleSort(int arr[], int n);
#endif
```
源文件 shell_bubble_sort.cpp:
```cpp
#include <iostream>
#include "shell_bubble_sort.h"
using namespace std;
void shellSort(int arr[], int n) {
int gap, i, j, temp;
int increments[] = {5, 3, 1};
int num_increments = 3;
for (int k = 0; k < num_increments; k++) {
gap = increments[k];
for (i = gap; i < n; i++) {
temp = arr[i];
for (j = i; j >= gap && arr[j - gap] > temp; j -= gap) {
arr[j] = arr[j - gap];
}
arr[j] = temp;
}
cout << "Shell Sort: ";
for (int k = 0; k < n; k++) {
cout << arr[k] << " ";
}
cout << endl;
}
}
void bubbleSort(int arr[], int n) {
int i, j, temp;
int num_rounds = n - 1;
for (int round = 1; round <= num_rounds; round++) {
for (i = 0; i < n - round; i++) {
if (arr[i] > arr[i + 1]) {
temp = arr[i];
arr[i] = arr[i + 1];
arr[i + 1] = temp;
}
}
cout << "Bubble Sort: ";
for (int k = 0; k < n; k++) {
cout << arr[k] << " ";
}
cout << endl;
}
}
```
主函数 main.cpp:
```cpp
#include <iostream>
#include "shell_bubble_sort.h"
using namespace std;
int main() {
int n;
cout << "Enter the size of the array: ";
cin >> n;
int arr[n];
cout << "Enter the elements of the array: ";
for (int i = 0; i < n; i++) {
cin >> arr[i];
}
shellSort(arr, n);
bubbleSort(arr, n);
return 0;
}
```
这个程序中,头文件 shell_bubble_sort.h 包含了两个函数的声明,源文件 shell_bubble_sort.cpp 实现了这两个函数,主函数 main.cpp 调用了这两个函数。在实现希尔排序时,增量数组为 {5, 3, 1},在实现冒泡排序时,比较轮次为 n - 1。程序运行时,会让用户输入数组长度和元素,然后进行排序,并在每趟排序后输出排序结果。
阅读全文
相关推荐
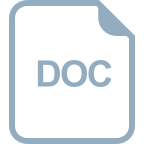
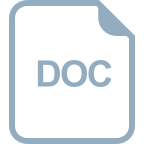
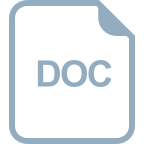





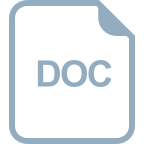
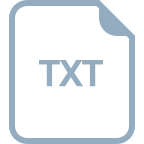
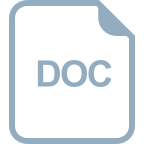
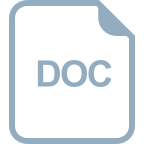
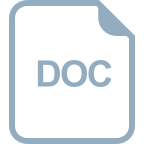
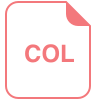




