screen = pygame.display.set_mode((SCREEN_WIDTH, SCREEN_HEIGHT))pygame.display.set_caption("Flappy Bird") ^^^^^^ SyntaxError: invalid syntax
时间: 2024-01-28 10:03:30 浏览: 36
这个错误的原因可能是你没有导入pygame模块,或者是导入的语句有误。请确保你已经正确安装了pygame模块,并且在你的代码中正确导入了它。正确的导入方式是:
```
import pygame
# 在这里编写你的代码
```
如果你已经正确导入了pygame模块,但是仍然出现这个错误,那么可能是你在其他部分的代码中出现了语法错误,导致这个错误提示出现在这一行。你可以检查一下你的代码,看看是否有其他的语法错误。
相关问题
# 初始化障碍物和柱子墙位置 obstacle_x = SCREEN_WIDTH pillar_x = [SCREEN_WIDTH + PILLAR_DISTANCE, SCREEN_WIDTH + PILLAR_DISTANCE * 2, SCREEN_WIDTH + PILLAR_DISTANCE * 3] # 游戏循环 while True: # 显示障碍物和柱子墙 draw_obstacle(obstacle_x, obstacle_y, obstacle_width, obstacle_height) for i in range(3): draw_pillar(pillar_x[i], pillar_height[i]) # 移动障碍物和柱子墙 obstacle_x -= OBSTACLE_SPEED for i in range(3): pillar_x[i] -= OBSTACLE_SPEED # 重新生成障碍物和柱子墙 if obstacle_x < -OBSTACLE_WIDTH: obstacle_x = SCREEN_WIDTH obstacle_y = random.randint(0, SCREEN_HEIGHT - OBSTACLE_HEIGHT) for i in range(3): if pillar_x[i] < -PILLAR_WIDTH: pillar_x[i] = SCREEN_WIDTH + PILLAR_DISTANCE * (i + 1) pillar_height[i] = random.randint(0, SCREEN_HEIGHT - PILLAR_GAP) # 初始化小鸟位置和速度 bird_x = BIRD_X bird_y = SCREEN_HEIGHT // 2 bird_v = 0 # 游戏循环 while True: # 监听用户输入 for event in pygame.event.get(): if event.type == pygame.KEYDOWN and event.key == pygame.K_SPACE: bird_v = BIRD_JUMP_SPEED # 移动小鸟 bird_y += bird_v bird_v += BIRD_GRAVITY # 碰撞检测 if bird_x + BIRD_WIDTH > obstacle_x and bird_x < obstacle_x + OBSTACLE_WIDTH \ and (bird_y < obstacle_y or bird_y + BIRD_HEIGHT > obstacle_y + OBSTACLE_HEIGHT): break for i in range(3): if bird_x + BIRD_WIDTH > pillar_x[i] and bird_x < pillar_x[i] + PILLAR_WIDTH \ and (bird_y < pillar_height[i] or bird_y + BIRD_HEIGHT > pillar_height[i] + PILLAR_GAP): break # 显示小鸟 draw_bird(bird_x, bird_y) # 游戏结束 if bird_y < 0 or bird_y + BIRD_HEIGHT > SCREEN_HEIGHT: break请帮我完善这段程序使其能在Python上运行不报错
这段程序看起来是一个使用 Pygame 库实现的小鸟飞跃柱子的游戏,但是缺少 Pygame 相关的初始化和显示代码。可以参考下面的代码进行补充:
```python
import pygame
import random
# 初始化 Pygame
pygame.init()
# 设置游戏窗口大小
SCREEN_WIDTH = 400
SCREEN_HEIGHT = 600
# 设置障碍物和柱子墙大小和间隔
OBSTACLE_WIDTH = 50
OBSTACLE_HEIGHT = 400
OBSTACLE_SPEED = 5
PILLAR_WIDTH = 50
PILLAR_GAP = 150
PILLAR_DISTANCE = 200
# 设置小鸟大小和速度
BIRD_WIDTH = 50
BIRD_HEIGHT = 50
BIRD_X = SCREEN_WIDTH // 2 - BIRD_WIDTH // 2
BIRD_JUMP_SPEED = -10
BIRD_GRAVITY = 1
# 初始化障碍物和柱子墙位置
obstacle_x = SCREEN_WIDTH
pillar_x = [SCREEN_WIDTH + PILLAR_DISTANCE, SCREEN_WIDTH + PILLAR_DISTANCE * 2, SCREEN_WIDTH + PILLAR_DISTANCE * 3]
obstacle_y = random.randint(0, SCREEN_HEIGHT - OBSTACLE_HEIGHT)
pillar_height = [random.randint(0, SCREEN_HEIGHT - PILLAR_GAP) for i in range(3)]
# 创建游戏窗口
screen = pygame.display.set_mode((SCREEN_WIDTH, SCREEN_HEIGHT))
pygame.display.set_caption("Flappy Bird")
# 显示障碍物和柱子墙
def draw_obstacle(x, y, w, h):
pygame.draw.rect(screen, (0, 255, 0), (x, y, w, h))
def draw_pillar(x, h):
pygame.draw.rect(screen, (0, 255, 0), (x, 0, PILLAR_WIDTH, h))
pygame.draw.rect(screen, (0, 255, 0), (x, h + PILLAR_GAP, PILLAR_WIDTH, SCREEN_HEIGHT - h - PILLAR_GAP))
# 显示小鸟
def draw_bird(x, y):
pygame.draw.rect(screen, (255, 0, 0), (x, y, BIRD_WIDTH, BIRD_HEIGHT))
# 初始化小鸟位置和速度
bird_x = BIRD_X
bird_y = SCREEN_HEIGHT // 2 - BIRD_HEIGHT // 2
bird_v = 0
# 游戏循环
while True:
# 监听用户输入
for event in pygame.event.get():
if event.type == pygame.QUIT:
pygame.quit()
exit()
elif event.type == pygame.KEYDOWN and event.key == pygame.K_SPACE:
bird_v = BIRD_JUMP_SPEED
# 显示背景
screen.fill((255, 255, 255))
# 显示障碍物和柱子墙
draw_obstacle(obstacle_x, obstacle_y, OBSTACLE_WIDTH, OBSTACLE_HEIGHT)
for i in range(3):
draw_pillar(pillar_x[i], pillar_height[i])
# 移动障碍物和柱子墙
obstacle_x -= OBSTACLE_SPEED
for i in range(3):
pillar_x[i] -= OBSTACLE_SPEED
# 重新生成障碍物和柱子墙
if obstacle_x < -OBSTACLE_WIDTH:
obstacle_x = SCREEN_WIDTH
obstacle_y = random.randint(0, SCREEN_HEIGHT - OBSTACLE_HEIGHT)
for i in range(3):
if pillar_x[i] < -PILLAR_WIDTH:
pillar_x[i] = SCREEN_WIDTH + PILLAR_DISTANCE * (i + 1)
pillar_height[i] = random.randint(0, SCREEN_HEIGHT - PILLAR_GAP)
# 移动小鸟
bird_y += bird_v
bird_v += BIRD_GRAVITY
# 碰撞检测
if bird_x + BIRD_WIDTH > obstacle_x and bird_x < obstacle_x + OBSTACLE_WIDTH \
and (bird_y < obstacle_y or bird_y + BIRD_HEIGHT > obstacle_y + OBSTACLE_HEIGHT):
break
for i in range(3):
if bird_x + BIRD_WIDTH > pillar_x[i] and bird_x < pillar_x[i] + PILLAR_WIDTH \
and (bird_y < pillar_height[i] or bird_y + BIRD_HEIGHT > pillar_height[i] + PILLAR_GAP):
break
# 显示小鸟
draw_bird(bird_x, bird_y)
# 游戏结束
if bird_y < 0 or bird_y + BIRD_HEIGHT > SCREEN_HEIGHT:
break
# 更新显示
pygame.display.update()
# 退出 Pygame
pygame.quit()
```
注意,这里的代码实现可能不完整或有错误,只能作为参考。如果还有问题,可以提出来一起讨论。
Flappy Bird环境代码
以下是使用Python和Pygame库编写的Flappy Bird环境代码:
```python
import pygame
import random
WIDTH = 288
HEIGHT = 512
FPS = 60
pygame.init()
pygame.mixer.init()
screen = pygame.display.set_mode((WIDTH, HEIGHT))
pygame.display.set_caption("Flappy Bird")
clock = pygame.time.Clock()
font_name = pygame.font.match_font('arial')
def draw_text(surf, text, size, x, y):
font = pygame.font.Font(font_name, size)
text_surface = font.render(text, True, (255, 255, 255))
text_rect = text_surface.get_rect()
text_rect.midtop = (x, y)
surf.blit(text_surface, text_rect)
def draw_base(base_x):
screen.blit(base_img, (base_x, 450))
screen.blit(base_img, (base_x + WIDTH, 450))
def draw_pipes(pipes):
for pipe in pipes:
if pipe.bottom >= 400:
screen.blit(pipe_img, (pipe.x, pipe.bottom))
else:
flip_pipe = pygame.transform.flip(pipe_img, False, True)
screen.blit(flip_pipe, (pipe.x, pipe.top))
def check_collision(player, pipes):
for pipe in pipes:
if pipe.top <= player.y <= pipe.bottom:
if pipe.x - 10 <= player.x <= pipe.x + 52:
return True
if player.y > 450 or player.y < 0:
return True
return False
class Player(pygame.sprite.Sprite):
def __init__(self):
pygame.sprite.Sprite.__init__(self)
self.image = pygame.image.load('assets/images/bird.png').convert_alpha()
self.rect = self.image.get_rect()
self.rect.centerx = 50
self.rect.centery = HEIGHT/2
self.y_speed = 0
def update(self):
self.y_speed += 1
self.rect.centery += self.y_speed
class Pipe:
def __init__(self):
self.top = random.randint(100, 300)
self.bottom = self.top + 150
self.x = WIDTH
def update(self):
self.x -= 2
def game():
player = Player()
pipes = []
score = 0
base_x = 0
pipe_timer = pygame.time.get_ticks()
running = True
while running:
clock.tick(FPS)
for event in pygame.event.get():
if event.type == pygame.QUIT:
running = False
if event.type == pygame.KEYDOWN:
if event.key == pygame.K_SPACE:
player.y_speed = -10
if pygame.time.get_ticks() - pipe_timer > 2000:
pipes.append(Pipe())
pipe_timer = pygame.time.get_ticks()
for pipe in pipes:
pipe.update()
if pipes and pipes[0].x < -50:
pipes.pop(0)
base_x -= 2
if base_x < -WIDTH:
base_x = 0
if check_collision(player, pipes):
running = False
for pipe in pipes:
if pipe.x == player.rect.centerx:
score += 1
screen.blit(background_img, (0, 0))
draw_pipes(pipes)
draw_base(base_x)
draw_text(screen, str(score), 32, WIDTH/2, 30)
player.update()
screen.blit(player.image, player.rect)
pygame.display.update()
pygame.quit()
background_img = pygame.image.load('assets/images/background.png').convert()
base_img = pygame.image.load('assets/images/base.png').convert()
pipe_img = pygame.image.load('assets/images/pipe.png').convert()
game()
```
这个代码实现了一个基本的Flappy Bird游戏环境,包括游戏逻辑、碰撞检测、得分计算等功能。你可以使用该环境来训练和测试自己编写的Flappy Bird AI算法。
相关推荐
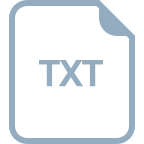
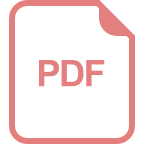









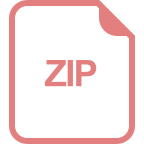
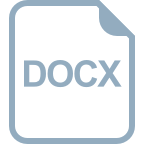