std::vector<std::vector<double>> transpose(std::vector<std::vector<double>> const arr) { int row = arr.size(); int col = arr[0].size(); // initialize transpose matrix col*row std::vector<std::vector<double>> trans(col); for(int i=0;i<col;i++){ trans[i].resize(row); } // fill elements for(int i=0; i<col;i++){ for(int j=0;j<row;j++){ trans[i][j] = arr[j][i]; } } return trans; }有错误吗
时间: 2024-03-06 15:50:00 浏览: 282
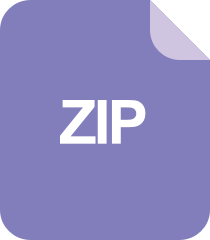
TransPose:转位
这个函数看起来是用于进行矩阵转置的,代码实现上没有明显的错误。不过,有一些地方可以改进:
1. 函数参数传递应该使用 const 引用,这样可以避免不必要的拷贝,提高代码效率。
2. 在初始化转置矩阵时,可以直接使用 `std::vector<std::vector<double>> trans(col, std::vector<double>(row));` 一行代码完成。
3. 在内层循环中,可以将行列交换一下,这样可以提高缓存命中率,从而提高代码效率。
下面是改进后的代码:
```
std::vector<std::vector<double>> transpose(std::vector<std::vector<double>> const& arr) {
int row = arr.size();
int col = arr[0].size();
// initialize transpose matrix col*row
std::vector<std::vector<double>> trans(col, std::vector<double>(row));
// fill elements
for(int j = 0; j < row; j++){
for(int i = 0; i < col; i++){
trans[i][j] = arr[j][i];
}
}
return trans;
}
```
阅读全文
相关推荐
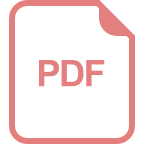

















