1. 某公司按周付给雇员报酬。公司有三种员工:钟点雇员,按小时付薪水,每周最多工作时间为40小时;试用雇员,每周固定薪水;佣金雇员,每周按销售额提成付给薪水。(2) Employee类中增加表示出生日期的属性birthday,birthday使用Date类型描述;增加出生日期的存取方法,(2) 增加行政人员类(Administrator),它公有继承自Employee,具有岗位工资(postWage)属性,设计必要的成员函数; (3) 增加销售经理类(MarketingManager),它公有继承自Administrator和CommissionEmployee,周薪水计算方式为岗位工资+销售提成,设计必要的成员函数;,用C++程序实现不同类型雇员薪水的计算。
时间: 2024-03-01 17:53:16 浏览: 36
好的,这个问题涉及到了几个类的设计和继承关系,需要一些代码实现。以下是一个基本的实现,您可以参考一下:
```c++
#include <iostream>
#include <string>
#include <vector>
#include <ctime>
using namespace std;
// Date类,用于存储日期
class Date {
public:
Date(int year, int month, int day) : year_(year), month_(month), day_(day) {}
int year() const { return year_; }
int month() const { return month_; }
int day() const { return day_; }
private:
int year_;
int month_;
int day_;
};
// Employee类,表示雇员
class Employee {
public:
Employee(string name, Date birthday) : name_(name), birthday_(birthday) {}
string name() const { return name_; }
Date birthday() const { return birthday_; }
virtual double salary() const = 0; // 纯虚函数,用于计算薪水
protected:
string name_;
Date birthday_;
};
// HourlyEmployee类,表示钟点雇员
class HourlyEmployee : public Employee {
public:
HourlyEmployee(string name, Date birthday, double hourly_wage, double max_work_hours)
: Employee(name, birthday), hourly_wage_(hourly_wage), max_work_hours_(max_work_hours) {}
double hourly_wage() const { return hourly_wage_; }
double max_work_hours() const { return max_work_hours_; }
double salary() const override {
double hours = min(work_hours_, max_work_hours_);
return hours * hourly_wage_;
}
void add_work_hours(double hours) {
work_hours_ += hours;
}
private:
double hourly_wage_;
double max_work_hours_;
double work_hours_ = 0.0;
};
// SalariedEmployee类,表示试用雇员
class SalariedEmployee : public Employee {
public:
SalariedEmployee(string name, Date birthday, double weekly_salary)
: Employee(name, birthday), weekly_salary_(weekly_salary) {}
double weekly_salary() const { return weekly_salary_; }
double salary() const override {
return weekly_salary_;
}
private:
double weekly_salary_;
};
// CommissionEmployee类,表示佣金雇员
class CommissionEmployee : public Employee {
public:
CommissionEmployee(string name, Date birthday, double commission_rate)
: Employee(name, birthday), commission_rate_(commission_rate) {}
double commission_rate() const { return commission_rate_; }
double salary() const override {
return sales_ * commission_rate_;
}
void add_sales(double sales) {
sales_ += sales;
}
private:
double commission_rate_;
double sales_ = 0.0;
};
// Administrator类,表示行政人员
class Administrator : public Employee {
public:
Administrator(string name, Date birthday, double post_wage)
: Employee(name, birthday), post_wage_(post_wage) {}
double post_wage() const { return post_wage_; }
double salary() const override {
return post_wage_;
}
private:
double post_wage_;
};
// MarketingManager类,表示销售经理
class MarketingManager : public Administrator, public CommissionEmployee {
public:
MarketingManager(string name, Date birthday, double post_wage, double commission_rate)
: Administrator(name, birthday, post_wage), CommissionEmployee(name, birthday, commission_rate) {}
double salary() const override {
return post_wage() + sales_ * commission_rate();
}
};
int main() {
// 创建雇员
HourlyEmployee hourly_employee("Tom", Date(1990, 1, 1), 10.0, 40.0);
SalariedEmployee salaried_employee("Jerry", Date(1995, 1, 1), 500.0);
CommissionEmployee commission_employee("Bob", Date(1998, 1, 1), 0.1);
MarketingManager marketing_manager("Alice", Date(1992, 1, 1), 1000.0, 0.2);
// 给雇员添加工作时间和销售额
hourly_employee.add_work_hours(50.0);
commission_employee.add_sales(2000.0);
marketing_manager.add_sales(5000.0);
// 计算雇员薪水
cout << hourly_employee.name() << "'s salary is " << hourly_employee.salary() << endl;
cout << salaried_employee.name() << "'s salary is " << salaried_employee.salary() << endl;
cout << commission_employee.name() << "'s salary is " << commission_employee.salary() << endl;
cout << marketing_manager.name() << "'s salary is " << marketing_manager.salary() << endl;
return 0;
}
```
此程序中,Employee类是一个抽象类,它包含一个纯虚函数salary(),用于计算薪水。HourlyEmployee、SalariedEmployee和CommissionEmployee类分别表示钟点雇员、试用雇员和佣金雇员,它们都是Employee的子类,并实现了自己的salary()函数。Administrator类表示行政人员,它公有继承自Employee,具有岗位工资(postWage)属性。MarketingManager类表示销售经理,它公有继承自Administrator和CommissionEmployee,周薪水计算方式为岗位工资+销售提成。
在主函数中,我们创建了四个不同类型的雇员,并给它们添加了工作时间和销售额,然后计算它们的薪水并输出。
相关推荐
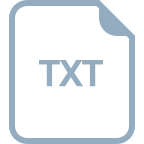
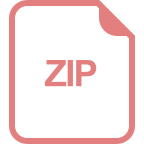
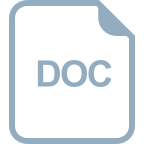














