class Population:
时间: 2023-10-03 16:04:31 浏览: 32
The Population class is a class that represents a group of individuals or organisms that share a specific set of characteristics or traits. This class typically contains methods for initializing, evaluating, and manipulating the population, as well as for selecting individuals for mating or reproduction. In the context of evolutionary algorithms, a Population class may also contain methods for performing genetic operations such as mutation and crossover, as well as for selecting individuals to be retained in the next generation.
相关问题
tmppar = GAhalf_Class(Population(randperm(end,2)));
这段代码的作用是从种群中随机选择两个体,并将它们为参数传递给`GAhalf_Class`函数,并将函数的返回值存在`tmppar`变量中。
具来说,`randperm(end,2)`表示生成一个随机的行向量,其中包含从1到`end`之间的整数,并且随机排序。这个行向量的长度为2,表示从种群中随机选择两个个体。
然后,`Population(randperm(end,2))`表示根据上一步生成的随机索引,从种群中选择对应的两个个体。
最后,这两个个体被作为参数传递给`GAhalf_Class`函数,并函数的返回值被赋值给`tmppar`变量。
Offspring = DE_Class(Parent1,Population(P(1)),Population(P(2)));
### 回答1:
这是一个差分进化算法(DE, Differential Evolution)中的操作,用于生成两个父代个体的交叉后的子代个体。其中Parent1表示从种群中选择的一个父代个体,Population(P(1))和Population(P(2))分别表示从种群中选择的另外两个个体。DE_Class函数是差分进化算法中的一个操作函数,它将两个父代个体进行差分操作,并将差分结果与第三个个体进行交叉操作,从而生成一个新的子代个体Offspring。具体来说,Offspring = DE_Class(Parent1,Population(P(1)),Population(P(2))),其中Offspring表示生成的子代个体。
### 回答2:
Offspring = DE_Class(Parent1, Population(P(1)), Population(P(2)));
这是一个数学表达式,用于表示子代的生成过程。
在这个表达式中,Offspring表示生成的子代。DE_Class是一个类或函数,可能是指差分进化算法相关的实现。Parent1是指父代中的某个个体,表示作为参考的基准个体。
Population(P(1))和Population(P(2))表示两个不同的个体群体,分别为P(1)和P(2)。这些个体可能是从父代中选择出来的一部分个体。
Offspring = DE_Class(Parent1, Population(P(1)), Population(P(2)))的含义是通过DE_Class算法,以Parent1为基准个体,结合P(1)和P(2)中的个体,来生成子代。具体的生成过程可能涉及到群体选择、交叉、变异等操作。
总之,这个表达式描述了一种使用差分进化算法生成子代的过程,其中父代中的一个个体作为参考,结合两个不同的个体群体,生成新的子代。
### 回答3:
这段代码是用来创建一个子类(offspring)。子类的名称是DE_Class,它继承自父类(Parent1)并包含两个成员变量(population(P(1))和population(P(2)))。
DE_Class是被称为子类或派生类的类,它继承了Parent1类的所有属性和方法。这意味着DE_Class可以使用父类中的成员变量和方法,同时也可以为其添加新的成员变量和方法。
population(P(1))和population(P(2))是DE_Class类的成员变量。它们可以是对象、数组等等。这些成员变量可以存储在DE_Class类的对象中,并在需要时被访问和操作。
这段代码将创建一个名为offspring的DE_Class类的实例。实例化一个类意味着在内存中为该类分配内存以及初始化其成员变量和方法。
综上所述,这段代码的含义是创建一个名为offspring的DE_Class类的实例,该子类继承自Parent1类,并包含两个成员变量population(P(1))和population(P(2))。这段代码实现了子类的创建和初始化。
相关推荐
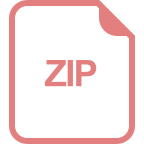
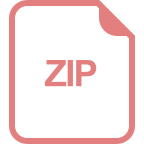
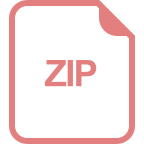










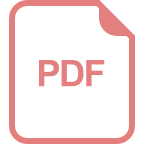
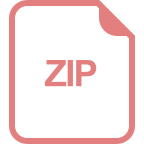
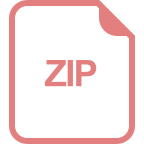