一百元钱买一百支笔,其中,钢笔3元一支,圆珠笔2元一支,铅笔0.5元一支c语言
时间: 2024-09-08 22:03:41 浏览: 65
在C语言中,你可以使用数组和循环结构来模拟这个购买情况。假设我们创建三个数组分别表示钢笔、圆珠笔和铅笔的数量,然后计算总价。以下是一个简单的示例:
```c
#include <stdio.h>
int main() {
int totalBills = 100; // 总金额
int steelPenCount = totalBills / 3; // 钢笔数量(因为每支3元)
int ballPenCount = totalBills / 2; // 圆珠笔数量(因为每支2元)
int pencilCount = totalBills * 2; // 铅笔数量(因为每个组合里有两支,共200支)
// 如果剩余金额不足以再买一支完整笔,则调整数量
if (totalBills % 3 > 0) {
steelPenCount += totalBills % 3;
}
if (totalBills % 2 > 0) {
ballPenCount += totalBills % 2;
}
float steelPenTotal = steelPenCount * 3; // 钢笔总费用
float ballPenTotal = ballPenCount * 2; // 圆珠笔总费用
printf("钢笔数量: %d 支, 总价: %.2f 元\n", steelPenCount, steelPenTotal);
printf("圆珠笔数量: %d 支, 总价: %.2f 元\n", ballPenCount, ballPenTotal);
// 假设所有剩下的就是铅笔
printf("铅笔数量: %d 支, 总价: %.2f 元\n", pencilCount - (steelPenCount + ballPenCount), pencilCount * 0.5);
return 0;
}
```
请注意,此代码只是一个简化版本,并未考虑到实际交易中可能存在的最小购买单位等限制。实际应用中,可能会需要更复杂的条件判断和数据结构。
阅读全文
相关推荐
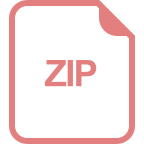
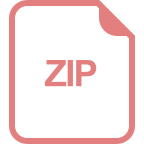
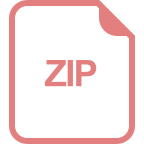





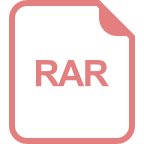
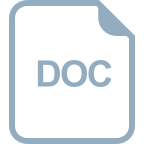
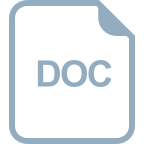
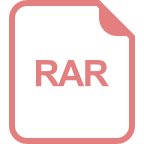





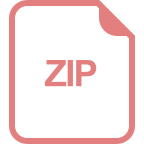