分支限界法求解旅行商计算最小出边费用
时间: 2025-01-04 21:24:34 浏览: 12
### 使用分支限界法求解旅行商问题 (TSP) 的最小出边费用
#### 定义与背景
旅行商问题 (Traveling Salesman Problem, TSP) 是组合优化中的经典难题之一。给定一组城市以及每对城市之间的距离,目标是找到一条最短路径使得访问每个城市恰好一次并返回起点。
对于寻找具有最小出边费用的解决方案,可以采用分支限界算法(branch and bound algorithm),这是一种通过剪枝来减少搜索空间的方法[^1]。
#### 分支限界法原理
该方法基于树形结构构建可能的路线,在遍历过程中不断评估部分解的成本,并利用界限条件排除那些不可能产生更优解的部分子树。具体到TSP中:
- **节点表示**:每一个节点代表当前已选的城市集合及其最后一个到达的城市。
- **边界函数**:用于估计完成剩余旅程所需的最低成本。这可以通过计算未访问城市的最小出度总和实现。
- **优先队列**:按照下界的升序排列待处理节点,从而总是先扩展最有潜力成为最优解的状态。
#### Python 实现示例
下面是一个简单的Python程序片段展示如何应用分支限界法解决带有最小出边约束的TSP:
```python
import heapq
from math import inf
def tsp_branch_and_bound(graph):
n = len(graph)
# 初始化起始状态
start_state = ([0], 0, 0) # 已访问过的城市列表(从索引0开始), 当前位置, 总花费
queue = []
best_cost = inf
optimal_path = None
def lower_bound(path, current_city, total_cost):
lb = total_cost
visited = set(path)
for i in range(n):
if i not in visited:
min_edge = min((graph[i][j] for j in range(n) if j != i and j not in visited), default=inf)
lb += min_edge
return lb
heapq.heappush(queue, (lower_bound(*start_state), start_state))
while queue:
_, (path, city, cost) = heapq.heappop(queue)
if len(path) == n:
last_to_first = graph[path[-1]][path[0]]
if cost + last_to_first < best_cost:
best_cost = cost + last_to_first
optimal_path = path[:] + [path[0]]
elif cost >= best_cost:
continue
else:
for next_city in range(n):
if next_city not in path:
new_path = path[:]
new_path.append(next_city)
travel_cost = graph[city][next_city]
heapq.heappush(
queue,
(
lower_bound(new_path, next_city, cost + travel_cost),
(new_path, next_city, cost + travel_cost)
)
)
return optimal_path, best_cost
# 测试数据集
test_graph = [
[0, 29, 20, 21],
[29, 0, 15, 17],
[20, 15, 0, 28],
[21, 17, 28, 0]
]
optimal_tour, tour_length = tsp_branch_and_bound(test_graph)
print(f"Optimal Tour: {optimal_tour}")
print(f"Tour Length: {tour_length}")
```
此代码实现了基本框架下的分支限界策略,其中`lower_bound()`函数负责提供必要的界限以指导搜索过程。注意这里假设输入图是以邻接矩阵形式给出的距离表。
阅读全文
相关推荐





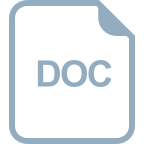












