[dict, avglen] = huffman(probabilities, symbols)写出在matlab能够运行的配套.m文件
时间: 2024-11-20 18:56:34 浏览: 5
在MATLAB中,Huffman编码是一种用于数据压缩的无损编码算法,它基于频率来构建哈夫曼树并生成对应的二进制码字。`[dict, avglen] = huffman(probabilities, symbols)`这段描述看起来像是函数调用,其中`probabilities`是一个包含字符概率分布的向量,`symbols`是一组需要编码的字符,`dict`将返回一个哈夫曼字典(映射每个字符到其编码),`avglen`则计算平均编码长度。
为了编写一个可以在MATLAB中运行的`huffman.m`文件,你可以参考以下结构:
```Matlab
function [dict, avgLen] = huffman(probabilities, symbols)
% HUFFMAN Huffman coding algorithm in MATLAB
% probabilities: Vector of character frequencies
% symbols: Characters to be encoded
% Ensure input types
if ~isnumeric(probabilities) || ~isscalar(probabilities) || length(probabilities) ~= length(symbols)
error('Invalid input: probabilities must be a scalar numeric vector of same length as symbols.');
end
% Create a priority queue for the Huffman tree construction
pq = PriorityQueue;
for i = 1:length(probabilities)
pq.insert([probabilities(i), i], symbols{i});
end
% Build the Huffman Tree and generate codes
while pq.size > 1
% Pop two smallest nodes from the queue
node1 = pq.removeMin();
node2 = pq.removeMin();
% Combine them into a new node with combined probability
newNode = Node(node1.probability + node2.probability, [node1, node2]);
pq.insert(newNode.probability, newNode);
end
% Convert the root node's path to a dictionary (mapping characters to codes)
root = pq.root;
dict = containers.Map();
traverse(root, '', dict);
% Calculate average code length
avgCodeLengths = cellfun(@(x) -x.probability * log2(x.probability), pq.nodes, 'UniformOutput', false);
avgLen = mean(avgCodeLengths);
% Helper function for traversing the Huffman tree
function traverse(node, prefix, dict)
if isLeaf(node)
dict(node.symbol) = prefix;
else
traverse(node.leftChild, prefix + '0', dict);
traverse(node.rightChild, prefix + '1', dict);
end
end
% Check if we have reached a leaf node
function isLeaf(node)
return isa(node, 'PriorityQueue.Node') && isequal(node.leftChild, []);
end
% Define a simple Node class (you may already have one in your toolbox)
classdef Node < handle
properties
probability
leftChild
rightChild
symbol
end
methods
% ... constructor and other methods here
end
end
end
```
这个`huffman.m`文件首先检查输入的有效性,然后构建哈夫曼树、生成编码字典,并计算平均编码长度。请注意,这里假设你已经有了`PriorityQueue`和`Node`类,如果没有,你需要自己实现它们。运行这个函数后,可以用`[dict, avgLen] = huffman(probabilities, symbols)`的形式来获取结果。
阅读全文
相关推荐
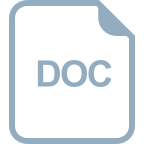
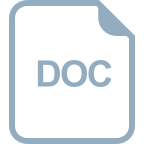
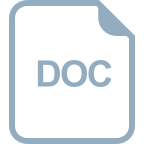















